-
-
Notifications
You must be signed in to change notification settings - Fork 1
Improve architecture and design principles #32
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
Merged
Merged
Changes from all commits
Commits
Show all changes
8 commits
Select commit
Hold shift + click to select a range
bd669ed
Improve architecture and design principles documentation
kamiazya cd7e145
Improve documentation structure
kamiazya d0f044b
Add Japanese translations and improve docs
kamiazya 3f4879e
chore: Fix for format
kamiazya ad569e8
chore: Refactor WebContainer component for build
kamiazya 01db8d5
chore: Improve docs
kamiazya fcd6008
doc: Improve package architecture documentation and add options for m…
kamiazya b09a573
chore: Alt description for dependency graph
kamiazya File filter
Filter by extension
Conversations
Failed to load comments.
Loading
Jump to
Jump to file
Failed to load files.
Loading
Diff view
Diff view
There are no files selected for viewing
File renamed without changes.
File renamed without changes.
File renamed without changes.
File renamed without changes.
File renamed without changes.
File renamed without changes
File renamed without changes
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
File renamed without changes.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,7 @@ | ||
{ | ||
"label": "Guides", | ||
"link": { | ||
"type": "generated-index", | ||
"description": "Guides to help you understand ts-graphviz." | ||
} | ||
} |
File renamed without changes
This file was deleted.
Oops, something went wrong.
34 changes: 34 additions & 0 deletions
34
docs/ts-graphviz/11-advanced-topics/01-design-principles.md
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,34 @@ | ||
--- | ||
description: Design principles of ts-graphviz. | ||
--- | ||
# Design Principles | ||
|
||
**ts-graphviz** is built around several key concepts that make it modular, extensible, and easy to use: | ||
|
||
1. **TypeScript-First Design & Type Definitions** | ||
|
||
**ts-graphviz** is designed primarily with TypeScript in mind, offering strong typing and seamless integration with TypeScript projects. Comprehensive type definitions for DOT language elements enable type-safe interactions, enhancing development efficiency and reducing errors. | ||
|
||
1. **Object-Oriented API** | ||
|
||
The library provides an object-oriented API for creating and manipulating graph elements such as graphs, nodes, and edges. This approach makes working with complex graph structures intuitive and efficient, leveraging familiar programming paradigms. | ||
|
||
1. **Modular Design** | ||
|
||
**ts-graphviz** adopts a modular architecture, split into multiple packages, each serving a specific purpose. This modularity allows users to include only the functionality they need, improving maintainability, flexibility, and reducing unnecessary dependencies. | ||
|
||
1. **AST Support** | ||
|
||
The library includes support for Abstract Syntax Trees (AST) for processing the DOT language. This enables parsing and generating DOT language while preserving its structure, facilitating programmatic manipulation and transformation of graphs. | ||
|
||
1. **Runtime Adapter** | ||
|
||
To ensure compatibility across different runtime environments, **ts-graphviz** provides adapter functions for environments like Node.js and Deno. These adapters serve as a wrapper, enabling seamless execution of Graphviz commands regardless of the underlying platform. | ||
|
||
1. **Extensibility** | ||
|
||
Designed with extensibility in mind, **ts-graphviz** allows users to extend its functionality with custom implementations for specific use cases. This flexibility supports a wide range of applications and integration scenarios. | ||
|
||
1. **Multi-Paradigm Support** | ||
|
||
The library accommodates various programming paradigms, including Object-Oriented Programming and Declarative Programming. Users can choose the style that best suits their needs, making the library versatile and adaptable. |
60 changes: 60 additions & 0 deletions
60
docs/ts-graphviz/11-advanced-topics/02-package-architecture.md
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,60 @@ | ||
--- | ||
description: Package architecture of ts-graphviz. | ||
--- | ||
# Package Architecture | ||
|
||
**ts-graphviz** consists of several packages, each with a specific role: | ||
|
||
- **`ts-graphviz`** (Main Package): Provides high-level APIs for creating and manipulating graphs, suitable for most users. | ||
|
||
- **`@ts-graphviz/core`**: Contains core implementations of models and functions, used internally and available for advanced use. | ||
|
||
- **`@ts-graphviz/common`**: Aggregates Graphviz domain knowledge, providing type definitions, constants, and utilities. Supports use cases like extending custom types and attributes. | ||
|
||
- **`@ts-graphviz/ast`**: Offers tools for parsing, manipulating, and generating DOT language graphs at the AST level. | ||
|
||
- **`@ts-graphviz/adapter`**: Executes Graphviz commands in various runtime environments (Node.js, Deno) and converts DOT strings to images. | ||
|
||
- **`@ts-graphviz/react`**: Allows defining graphs using React's declarative UI paradigm, expressing DOT language models with JSX. | ||
|
||
## Exposing Internal Modules as Submodules | ||
|
||
**ts-graphviz** publishes some of its internal modules as submodules within the main package. Specifically, the following import paths are available to access these internal modules: | ||
|
||
- **`ts-graphviz/ast`** or **`@ts-graphviz/ast`** | ||
- **`ts-graphviz/adapter`** or **`@ts-graphviz/adapter`** | ||
|
||
This allows users to choose the module import style that best fits their project's needs. By using the `ts-graphviz/<module-name>` style, you can limit your dependencies to the `ts-graphviz` library itself, with internal packages (e.g., `@ts-graphviz/adapter`, `@ts-graphviz/ast`) being managed centrally by the `ts-graphviz` package. On the other hand, using the `@ts-graphviz/<module-name>` style enables you to manage dependencies on a per-module basis, allowing you to selectively install specific modules as needed. | ||
|
||
:::tip Managing Dependencies When Choosing Import Paths | ||
|
||
When using package managers that enforce strict dependency management (e.g., pnpm), the choice of import path can significantly impact how dependencies are handled. Consider the following points: | ||
|
||
- **Using `ts-graphviz/<module-name>` Style**: | ||
- **Advantages**: Limits dependencies to the `ts-graphviz` library itself, with internal packages (e.g., `@ts-graphviz/adapter`, `@ts-graphviz/ast`) being managed centrally by the `ts-graphviz` package. This prevents dependency conflicts and ensures that stable versions recommended by the library are used. | ||
- **Recommended Scenarios**: When you want to minimize dependencies or when the internal packages managed by `ts-graphviz` do not have specific version requirements. | ||
|
||
- **Using `@ts-graphviz/<module-name>` Style**: | ||
- **Advantages**: Allows you to manage dependencies on a per-module basis, installing only the specific modules you need, which can help keep your application lightweight. | ||
- **Caveats**: Requires you to manage internal dependencies (e.g., `@ts-graphviz/adapter`, `@ts-graphviz/ast`) separately within your package manager. This can complicate version management. | ||
- **Recommended Scenarios**: When you need to use specific modules only or require detailed control over your dependencies. | ||
|
||
**Summary**: | ||
- If you prefer **simple dependency management**, use the `ts-graphviz/<module-name>` style. | ||
- If you require **fine-grained control over dependencies** or only need specific modules, opt for the `@ts-graphviz/<module-name>` style. | ||
|
||
This approach allows you to manage dependencies in a way that best suits your project's requirements. | ||
::: | ||
|
||
|
||
The relationships between packages can be visualized as follows: | ||
|
||
 | ||
|
||
This modular architecture ensures: | ||
|
||
- **Maintainability**: Individual packages can be maintained and updated without affecting others. | ||
|
||
- **Flexibility**: Users can select only the packages needed for their specific use cases. | ||
|
||
- **Extensibility**: Facilitates the addition of new features or packages as the library evolves. |
49 changes: 49 additions & 0 deletions
49
docs/ts-graphviz/11-advanced-topics/03-versioning-policy.md
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,49 @@ | ||
--- | ||
description: Versioning policy for ts-graphviz. | ||
--- | ||
# Versioning Policy | ||
|
||
## Overview | ||
|
||
**ts-graphviz** follows [**Semantic Versioning 2.0**](https://semver.org/) to ensure consistency and predictability. This policy clarifies how we manage breaking changes, especially those related to runtime support, and what users can expect when using **ts-graphviz**. | ||
|
||
## Semantic Versioning | ||
|
||
Version numbers are formatted as **MAJOR.MINOR.PATCH**, with changes classified as follows: | ||
|
||
- **MAJOR**: Introduces backward-incompatible changes. | ||
- **MINOR**: Adds functionality in a backward-compatible manner. | ||
- **PATCH**: Fixes bugs in a backward-compatible manner. | ||
|
||
--- | ||
|
||
## Exceptions: TypeScript Version Updates | ||
|
||
There are scenarios where **ts-graphviz** may deviate from strict Semantic Versioning: | ||
|
||
- **TypeScript Definitions**: Breaking changes to TypeScript type definitions may occur between minor versions due to: | ||
- TypeScript introducing breaking changes in its own minor updates. | ||
- Adopting features available only in newer TypeScript versions, which may raise the minimum required TypeScript version. | ||
|
||
:::tip | ||
For TypeScript projects, we recommend pinning the **ts-graphviz** minor version to control upgrade timing and compatibility testing. | ||
::: | ||
|
||
--- | ||
|
||
## Information Sharing | ||
|
||
We are committed to transparent communication regarding our versioning and support policies: | ||
|
||
- **Documentation and Release Notes**: All changes to the versioning policy and support levels will be documented in our official documentation and detailed in release notes. | ||
- **Community Engagement**: Users are encouraged to subscribe to updates or follow our repositories to stay informed about the latest developments. | ||
|
||
--- | ||
|
||
## Migration Support | ||
|
||
To assist users in transitioning between versions, especially when breaking changes occur: | ||
|
||
- **Migration Guides**: We provide comprehensive migration guides outlining steps to upgrade to new major versions. | ||
- **Code Examples**: Examples are included to demonstrate how to adapt code to accommodate changes. | ||
- **Support Channels**: Users can seek assistance through community forums, issue trackers, or other support channels if they encounter difficulties during migration. |
44 changes: 44 additions & 0 deletions
44
docs/ts-graphviz/11-advanced-topics/04-supported-environments.md
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,44 @@ | ||
--- | ||
description: Supported environments for ts-graphviz. | ||
draft: true | ||
--- | ||
# Supported Environments | ||
|
||
To provide clarity on the environments in which **ts-graphviz** operates, we have categorized support levels: | ||
|
||
## Support Levels | ||
|
||
### Tier 1: Full Support | ||
|
||
- **Definition**: Environments that are fully supported, with comprehensive automated testing and maintenance. | ||
- **Environments**: | ||
- **Node.js**: All active Long-Term Support (LTS) versions. | ||
- **Details**: | ||
- We run automated tests on all LTS versions of Node.js. | ||
- Full compatibility and performance are ensured. | ||
- Critical issues are prioritized for fixes. | ||
|
||
### Tier 2: Active Support | ||
|
||
- **Definition**: Environments that receive active support with limited automated testing. | ||
- **Environments**: | ||
- **Deno**: Latest stable version. | ||
- **Node.js Current Release**: The latest Node.js release outside the LTS schedule. | ||
- **Details**: | ||
- Automated tests are conducted on the latest version of Deno to confirm functionality. | ||
- Compatibility is maintained, and issues are addressed. | ||
|
||
### Tier 3: Community Support | ||
|
||
- **Definition**: Environments that are not officially tested but are supported on a best-effort basis. | ||
- **Environments**: | ||
- **Modern Browsers**: Latest versions of major browsers, including: | ||
- Google Chrome | ||
- Mozilla Firefox | ||
- Microsoft Edge | ||
- Apple Safari | ||
- **Details**: | ||
- Installation methods are provided. | ||
- No automated testing is performed. | ||
- Issues reported by users will be addressed. | ||
- Targeting the latest versions ensures compatibility with modern web standards. |
34 changes: 34 additions & 0 deletions
34
docs/ts-graphviz/11-advanced-topics/05-security-policies.md
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,34 @@ | ||
--- | ||
description: Security policies for ts-graphviz. | ||
--- | ||
# Security Policies | ||
|
||
## Purpose and Importance | ||
|
||
In today's software development landscape, **Open Source Software (OSS)** plays an indispensable role in building applications. According to the **Open Source Security Foundation (OpenSSF)**, **70% to 90%** of modern applications consist of OSS components[^1], and the complexity of their dependencies is increasing daily. | ||
|
||
[^1]: Behlendorf, B. (2022, May 11). *Brian Behlendorf Testifies to Congress on Open Source Software Security* (original in English). The Linux Foundation. Retrieved from [https://www.linuxfoundation.org/blog/blog/lf/brian-behlendorf-testifies-open-source-software-security](https://www.linuxfoundation.org/blog/blog/lf/brian-behlendorf-testifies-open-source-software-security) | ||
|
||
### The Impact and Responsibility of ts-graphviz | ||
|
||
**ts-graphviz** is a widely used library with over 2 million downloads per month, impacting a broad range of applications. Even users who do not directly interact with ts-graphviz are affected through software that depends on it. | ||
|
||
> 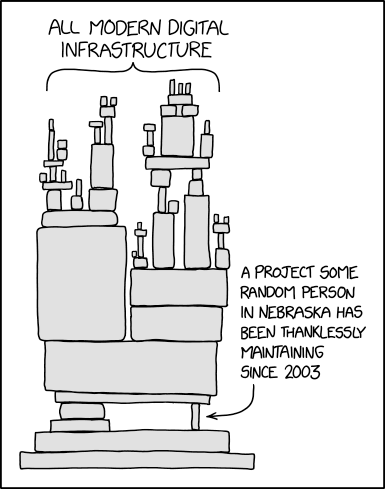 | ||
> | ||
> *An illustration of software dependencies (Source: [xkcd.com/2347](https://xkcd.com/2347/))* | ||
|
||
This illustration highlights how modern software relies on numerous underlying components. | ||
|
||
**Ensuring the security of ts-graphviz is crucial because vulnerabilities can cascade through dependencies, potentially affecting countless applications.** | ||
|
||
## Commitment to Security | ||
|
||
ts-graphviz is committed to more than just providing secure source code. We implement **comprehensive security measures** that consider the **entire software supply chain**. This dedication ensures that users can confidently adopt ts-graphviz, knowing it contributes to building secure and reliable applications. | ||
|
||
## Software Supply Chain Security | ||
|
||
Recognizing that modern applications are heavily dependent on open-source software, we implement practices that safeguard against vulnerabilities throughout the dependency chain. | ||
|
||
## Reporting Vulnerabilities | ||
|
||
We encourage users and security researchers to report any vulnerabilities or security concerns. Prompt reporting allows us to address issues swiftly, maintaining the integrity and security of the library. | ||
kamiazya marked this conversation as resolved.
Show resolved
Hide resolved
|
3 changes: 1 addition & 2 deletions
3
...raphviz/11-advanced-usage/_category_.json → ...aphviz/11-advanced-topics/_category_.json
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -1,8 +1,7 @@ | ||
{ | ||
"label": "Advanced Usage", | ||
"label": "Advanced Topics", | ||
"link": { | ||
"type": "generated-index", | ||
"description": "Advanced topics to help you understand the inner workings of ts-graphviz." | ||
} | ||
} | ||
|
File renamed without changes
Oops, something went wrong.
Oops, something went wrong.
Add this suggestion to a batch that can be applied as a single commit.
This suggestion is invalid because no changes were made to the code.
Suggestions cannot be applied while the pull request is closed.
Suggestions cannot be applied while viewing a subset of changes.
Only one suggestion per line can be applied in a batch.
Add this suggestion to a batch that can be applied as a single commit.
Applying suggestions on deleted lines is not supported.
You must change the existing code in this line in order to create a valid suggestion.
Outdated suggestions cannot be applied.
This suggestion has been applied or marked resolved.
Suggestions cannot be applied from pending reviews.
Suggestions cannot be applied on multi-line comments.
Suggestions cannot be applied while the pull request is queued to merge.
Suggestion cannot be applied right now. Please check back later.
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
💡 Codebase verification
Based on the search results from the documentation and package.json files, I can now provide a final response:
Documentation needs to be updated to include all available submodules
The documentation should be updated to include all four available submodules:
ts-graphviz/ast
or@ts-graphviz/ast
ts-graphviz/adapter
or@ts-graphviz/adapter
ts-graphviz/common
or@ts-graphviz/common
ts-graphviz/core
or@ts-graphviz/core
Evidence:
@ts-graphviz/adapter
,@ts-graphviz/ast
,@ts-graphviz/common
, and@ts-graphviz/core
import { $keywords } from '@ts-graphviz/common'
ts-graphviz/ast
and@ts-graphviz/ast
)🔗 Analysis chain
Verify completeness of available submodules.
Please verify if
common
andcore
packages are also available as submodules. If they are, they should be listed alongsideast
andadapter
.Let's check the package.json and exports configuration:
🏁 Scripts executed
The following scripts were executed for the analysis:
Script:
Length of output: 143
Script:
Length of output: 2712
Script:
Length of output: 406
Script:
Length of output: 14923
🧰 Tools
🪛 LanguageTool
[duplication] ~24-~24: Possible typo: you repeated a word
Context: ...ernal modules: -
ts-graphviz/ast
or@ts-graphviz/ast
-ts-graphviz/adapter
or@ts-graphviz/adapter
This allows...(ENGLISH_WORD_REPEAT_RULE)
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
@ts-graphviz/common
and@ts-graphviz/core
are not public.There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
@kamiazya, understood. Thanks for clarifying that
@ts-graphviz/common
and@ts-graphviz/core
are not public. We’ll keep that in mind when documenting the submodules. Please let me know if there’s anything else you’d like to adjust or clarify.✏️ Learnings added