-
Notifications
You must be signed in to change notification settings - Fork 2
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
Fast Forward.... 4 years later... (#13)
* hopefully more accurate * some spellchecking and cleaning, also fixing CLI * fixed whitespace * testing new functionality for motor drivers and automatic current setting * squashing bugs * bug fixin * no functionality added, just cleaning code * added sensor reset for magnetometer * works pretty well, needs some control theory and finishing touches * hopefully didn't break anything, hopefully made system faster/more responsive * Updated Updated with previous edits to remove unnecessary branches. * Added Arduino Code and README Added new folder that includes code and README description for Arduino. The purpose is for the Arduino to interact with the magnetometer. --------- Co-authored-by: PandnotPthereforeQ <[email protected]> Co-authored-by: jejor <[email protected]> Co-authored-by: Cordet <[email protected]>
- Loading branch information
1 parent
5fcdc16
commit 652c9e6
Showing
13 changed files
with
386 additions
and
109 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
|
@@ -11,6 +11,7 @@ __pycache__/ | |
*.so | ||
|
||
# Distribution / packaging | ||
.vscode | ||
.Python | ||
build/ | ||
develop-eggs/ | ||
|
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,49 @@ | ||
COMMS_README.TXT | ||
|
||
This document is the decode key to "ArduinoComms.py" and "PSAS_HHCage.ino" | ||
|
||
General: | ||
The arduino will initialize the magnetic sensor (if present) and listen on USB-Serial (115200) for one byte messages from the Raspberry PI / Python. The Arduino will control 3 H-bridges (X,Y,Z) and interact with a magnetometer (MMC5603). Some commands from the PI will recieve a serial data return. | ||
|
||
Note that decode values are case sensitive.The following are the commands the arduino is listening for without any serial data returned: | ||
x - Activate X H-bridge in Positive Polarity | ||
y - Activate Y H-bridge in Positive Polarity | ||
z - Activate Z H-bridge in Positive Polarity | ||
X - Activate X H-bridge in Negative Polarity | ||
Y - Activate Y H-bridge in Negative Polarity | ||
Z - Activate Z H-bridge in Negative Polarity | ||
a - De-activate all H-Bridges (X,Y,Z) | ||
b - De-activate X H-Bridge | ||
c - De-activate Y H-Bridge | ||
d - De-activate Z H-Bridge | ||
The following are the commands the arduino is listening for with a serial data return: | ||
m - Request current magnetic field reading | ||
Data return is "X,Y,Z" magnetic field in uT. | ||
The values of each value X,Y,Z can be positive or negative | ||
Here is an example return: "1000.05,-200.33,500.79" | ||
Note: Refer to the nomen on the magnetometer to interpret positive and negative field directions | ||
q - Request magnetometer status | ||
Data return is "0" -- magnetometer not initialized | ||
"1" -- magnetometer is initialized | ||
note: magnetometer is initialized on setup/startup of arduino script. Restarting the serial interface will reset the arduino and will attempt re-initialization. If failures persist inspect wiring to sensor and the physical sensor. | ||
s - Request H-Bridge Status: | ||
Data return is "XYZ" where | ||
X is X axis H-Bridge status | ||
Y is Y axis H-Bridge status | ||
Z is Z axis H-Bridge status | ||
Each position can be 0, 1, or 2: | ||
0: Bridge is de-activated | ||
1: Bridge is activated in positive polarity | ||
2: Bridge is activated in negative polarity | ||
Example: | ||
021 | ||
X axis H-Bridge is de-activated | ||
Y axis H-Bridge is activated in negative polarity | ||
Z axis H-Bridge is activated in positive polarity | ||
t - Request Ambient Temperature from Magnetometer: | ||
The magnetometer has a temperature sensor built in, might as well provide the ability to read it. | ||
The Serial data return is in degrees Celsius: | ||
##.## | ||
Example: 17.80 | ||
|
||
# Credit to Christian Bennett (2022) for assisting with Arduino code and testing |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,154 @@ | ||
#include <Adafruit_MMC56x3.h> | ||
/* Assign a unique ID to this sensor at the same time */ | ||
Adafruit_MMC5603 mmc = Adafruit_MMC5603(12345); | ||
|
||
int xina = 6; | ||
int xinb = 7; | ||
int yina = 4; | ||
int yinb = 5; | ||
int zina = 2; | ||
int zinb = 3; | ||
int xstat = 0; | ||
int ystat = 0; | ||
int zstat = 0; | ||
int magstat = 0; | ||
|
||
|
||
int incomingByte = 0; // for incoming serial data | ||
|
||
void setup() { | ||
// put your setup code here, to run once: | ||
pinMode(xina, OUTPUT); | ||
pinMode(xinb, OUTPUT); | ||
pinMode(yina, OUTPUT); | ||
pinMode(yinb, OUTPUT); | ||
pinMode(zina, OUTPUT); | ||
pinMode(zinb, OUTPUT); | ||
digitalWrite(xina,LOW); | ||
digitalWrite(xinb,LOW); | ||
digitalWrite(yina,LOW); | ||
digitalWrite(yinb,LOW); | ||
digitalWrite(zina,LOW); | ||
digitalWrite(zinb,LOW); | ||
Serial.begin(115200); | ||
|
||
|
||
// Initialise the mag sensor */ | ||
if (mmc.begin(MMC56X3_DEFAULT_ADDRESS, &Wire)) { // I2C mode | ||
magstat = 1; | ||
//mmc.printSensorDetails(); | ||
} | ||
|
||
} | ||
|
||
void loop() { | ||
// put your main code here, to run repeatedly: | ||
|
||
// reply only when you receive data: | ||
if (Serial.available() > 0) { | ||
// read the incoming byte: | ||
incomingByte = Serial.read(); | ||
|
||
// say what you got: | ||
// Serial.print("I received: "); | ||
// Serial.println(incomingByte, DEC); | ||
} | ||
if (incomingByte != 0){ | ||
//Shutdown | ||
if (incomingByte == 97){ | ||
digitalWrite(xina,LOW); | ||
digitalWrite(xinb,LOW); | ||
digitalWrite(yina,LOW); | ||
digitalWrite(yinb,LOW); | ||
digitalWrite(zina,LOW); | ||
digitalWrite(zinb,LOW); | ||
xstat = 0; | ||
ystat = 0; | ||
zstat = 0; | ||
} | ||
//X Bridge Off | ||
if (incomingByte == 98){ | ||
digitalWrite(xina,LOW); | ||
digitalWrite(xinb,LOW); | ||
xstat = 0; | ||
} | ||
//Y Bridge Off | ||
if (incomingByte == 99){ | ||
digitalWrite(yina,LOW); | ||
digitalWrite(yinb,LOW); | ||
ystat = 0; | ||
} | ||
//Z Bridge Off | ||
if (incomingByte == 100){ | ||
digitalWrite(zina,LOW); | ||
digitalWrite(zinb,LOW); | ||
zstat = 0; | ||
} | ||
//H-Bridge Status message | ||
if (incomingByte == 115){ | ||
Serial.print(xstat); | ||
Serial.print(ystat); | ||
Serial.println(zstat); | ||
} | ||
//mag Status message | ||
if (incomingByte == 113){ | ||
Serial.println(magstat); | ||
} | ||
//positive "x" | ||
if (incomingByte == 120){ | ||
digitalWrite(xinb,LOW); | ||
digitalWrite(xina,HIGH); | ||
xstat = 1; | ||
} | ||
//negative "X" | ||
if (incomingByte == 88){ | ||
digitalWrite(xina,LOW); | ||
digitalWrite(xinb,HIGH); | ||
xstat = 2; | ||
} | ||
//positive "y" | ||
if (incomingByte == 121){ | ||
digitalWrite(yinb,LOW); | ||
digitalWrite(yina,HIGH); | ||
ystat = 1; | ||
} | ||
//negative "Y" | ||
if (incomingByte == 89){ | ||
digitalWrite(yina,LOW); | ||
digitalWrite(yinb,HIGH); | ||
ystat = 2; | ||
} | ||
//positive "z" | ||
if (incomingByte == 122){ | ||
digitalWrite(zinb,LOW); | ||
digitalWrite(zina,HIGH); | ||
zstat = 1; | ||
} | ||
//negative "Z" | ||
if (incomingByte == 90){ | ||
digitalWrite(zina,LOW); | ||
digitalWrite(zinb,HIGH); | ||
zstat = 2; | ||
} | ||
//mag reading | ||
if (incomingByte == 109){ | ||
sensors_event_t event; | ||
mmc.getEvent(&event); | ||
Serial.print(event.magnetic.x); | ||
Serial.print(","); | ||
Serial.print(event.magnetic.y); | ||
Serial.print(","); | ||
Serial.println(event.magnetic.z); | ||
} | ||
//temp reading | ||
if (incomingByte == 116){ | ||
sensors_event_t event; | ||
mmc.getEvent(&event); | ||
float temp_c = mmc.readTemperature(); | ||
Serial.println(temp_c); | ||
} | ||
//end of loop | ||
incomingByte = 0; | ||
Serial.flush(); | ||
} | ||
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -1,11 +1,11 @@ | ||
## oresat-helmholtz | ||
# oresat-helmholtz | ||
|
||
 | ||
|
||
## Magnetic Environment Simulator for CubeSats | ||
## Magnetic Environment Simulator for CubeSats | ||
|
||
SOP can be found [here](http://psu-epl.github.io/doc/equip/testing/ETL/) at the Electronics Prototyping Lab website | ||
|
||
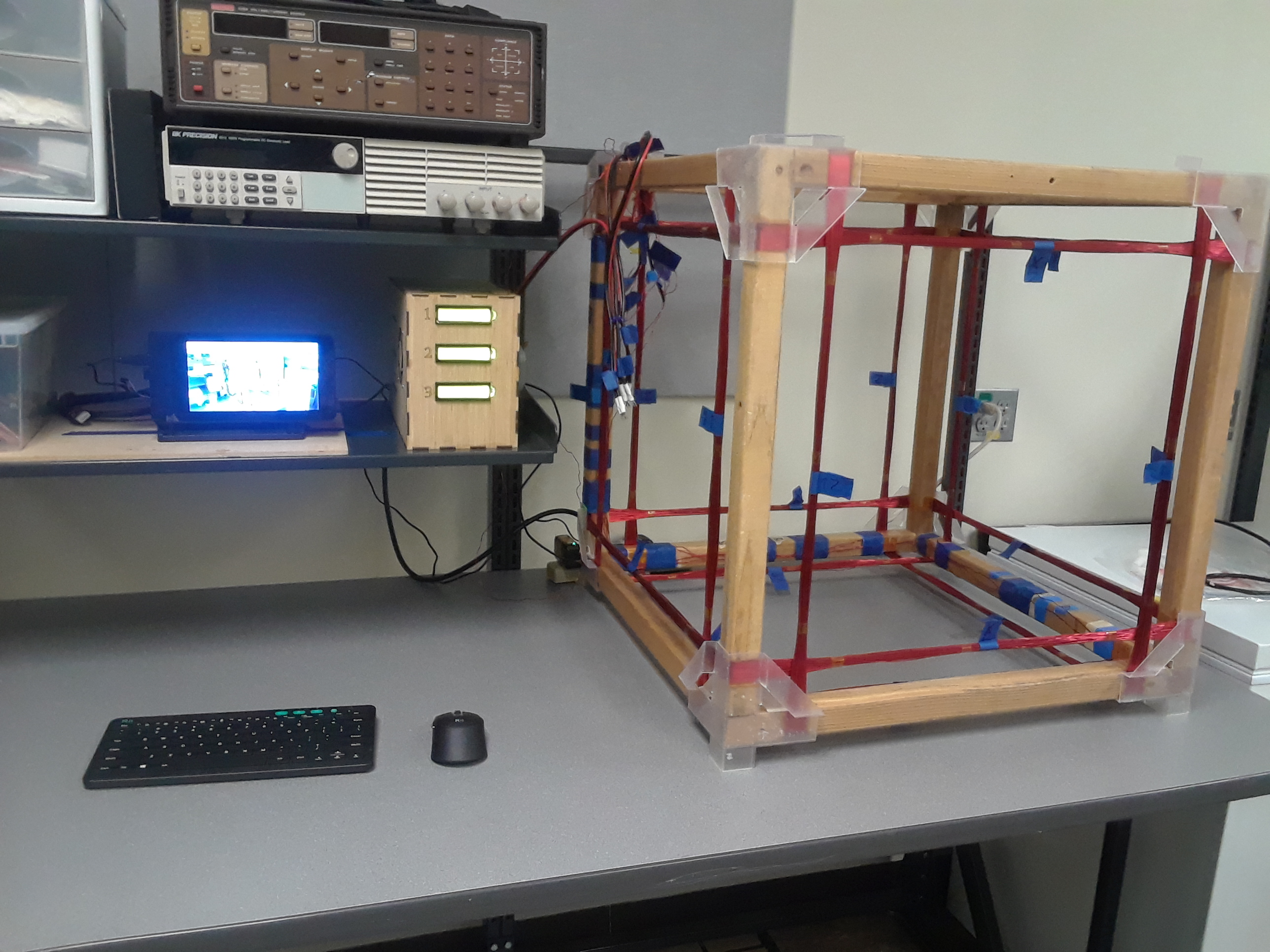 | ||
|
||
|
||
MCECS BETA Project 2018 |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Oops, something went wrong.