Commit ead8510
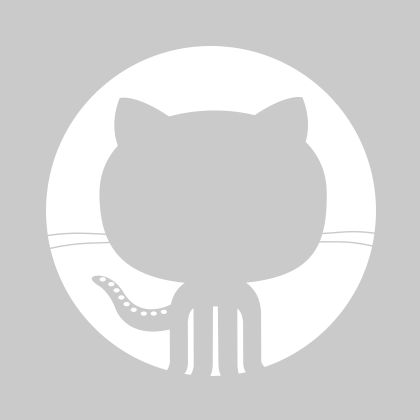
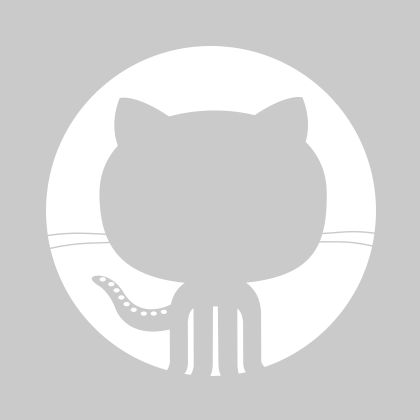
Charlie Frasch
Charlie Frasch
1 parent 17e8c63 commit ead8510
File tree
13 files changed
+545
-83
lines changed13 files changed
+545
-83
lines changedOriginal file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
41 | 41 |
| |
42 | 42 |
| |
43 | 43 |
| |
| 44 | + | |
44 | 45 |
| |
| 46 | + | |
45 | 47 |
| |
46 | 48 |
| |
47 | 49 |
| |
|
+7-4
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
23 | 23 |
| |
24 | 24 |
| |
25 | 25 |
| |
26 |
| - | |
| 26 | + | |
27 | 27 |
| |
28 | 28 |
| |
29 | 29 |
| |
| |||
57 | 57 |
| |
58 | 58 |
| |
59 | 59 |
| |
60 |
| - | |
| 60 | + | |
61 | 61 |
| |
62 | 62 |
| |
63 | 63 |
| |
| |||
70 | 70 |
| |
71 | 71 |
| |
72 | 72 |
| |
73 |
| - | |
74 |
| - | |
| 73 | + | |
| 74 | + | |
75 | 75 |
| |
76 | 76 |
| |
77 | 77 |
| |
| |||
83 | 83 |
| |
84 | 84 |
| |
85 | 85 |
| |
| 86 | + | |
| 87 | + | |
| 88 | + | |
86 | 89 |
| |
87 | 90 |
| |
88 | 91 |
| |
|
+13-10
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
23 | 23 |
| |
24 | 24 |
| |
25 | 25 |
| |
26 |
| - | |
| 26 | + | |
27 | 27 |
| |
28 | 28 |
| |
29 | 29 |
| |
| |||
54 | 54 |
| |
55 | 55 |
| |
56 | 56 |
| |
57 |
| - | |
58 |
| - | |
59 |
| - | |
| 57 | + | |
| 58 | + | |
| 59 | + | |
60 | 60 |
| |
61 | 61 |
| |
62 |
| - | |
| 62 | + | |
63 | 63 |
| |
64 | 64 |
| |
65 | 65 |
| |
| |||
70 | 70 |
| |
71 | 71 |
| |
72 | 72 |
| |
73 |
| - | |
74 |
| - | |
75 |
| - | |
| 73 | + | |
| 74 | + | |
| 75 | + | |
76 | 76 |
| |
77 | 77 |
| |
78 |
| - | |
79 |
| - | |
| 78 | + | |
| 79 | + | |
80 | 80 |
| |
81 | 81 |
| |
82 | 82 |
| |
| |||
88 | 88 |
| |
89 | 89 |
| |
90 | 90 |
| |
| 91 | + | |
| 92 | + | |
| 93 | + | |
91 | 94 |
| |
92 | 95 |
| |
93 | 96 |
| |
|
+14-11
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
23 | 23 |
| |
24 | 24 |
| |
25 | 25 |
| |
26 |
| - | |
| 26 | + | |
27 | 27 |
| |
28 | 28 |
| |
29 | 29 |
| |
| |||
41 | 41 |
| |
42 | 42 |
| |
43 | 43 |
| |
44 |
| - | |
| 44 | + | |
45 | 45 |
| |
46 | 46 |
| |
47 | 47 |
| |
| |||
54 | 54 |
| |
55 | 55 |
| |
56 | 56 |
| |
57 |
| - | |
58 |
| - | |
59 |
| - | |
| 57 | + | |
| 58 | + | |
| 59 | + | |
60 | 60 |
| |
61 | 61 |
| |
62 |
| - | |
| 62 | + | |
63 | 63 |
| |
64 | 64 |
| |
65 | 65 |
| |
| |||
70 | 70 |
| |
71 | 71 |
| |
72 | 72 |
| |
73 |
| - | |
74 |
| - | |
75 |
| - | |
| 73 | + | |
| 74 | + | |
| 75 | + | |
76 | 76 |
| |
77 | 77 |
| |
78 |
| - | |
79 |
| - | |
| 78 | + | |
| 79 | + | |
80 | 80 |
| |
81 | 81 |
| |
82 | 82 |
| |
| |||
88 | 88 |
| |
89 | 89 |
| |
90 | 90 |
| |
| 91 | + | |
| 92 | + | |
| 93 | + | |
91 | 94 |
| |
92 | 95 |
| |
93 | 96 |
| |
|
+126
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
| 1 | + | |
| 2 | + | |
| 3 | + | |
| 4 | + | |
| 5 | + | |
| 6 | + | |
| 7 | + | |
| 8 | + | |
| 9 | + | |
| 10 | + | |
| 11 | + | |
| 12 | + | |
| 13 | + | |
| 14 | + | |
| 15 | + | |
| 16 | + | |
| 17 | + | |
| 18 | + | |
| 19 | + | |
| 20 | + | |
| 21 | + | |
| 22 | + | |
| 23 | + | |
| 24 | + | |
| 25 | + | |
| 26 | + | |
| 27 | + | |
| 28 | + | |
| 29 | + | |
| 30 | + | |
| 31 | + | |
| 32 | + | |
| 33 | + | |
| 34 | + | |
| 35 | + | |
| 36 | + | |
| 37 | + | |
| 38 | + | |
| 39 | + | |
| 40 | + | |
| 41 | + | |
| 42 | + | |
| 43 | + | |
| 44 | + | |
| 45 | + | |
| 46 | + | |
| 47 | + | |
| 48 | + | |
| 49 | + | |
| 50 | + | |
| 51 | + | |
| 52 | + | |
| 53 | + | |
| 54 | + | |
| 55 | + | |
| 56 | + | |
| 57 | + | |
| 58 | + | |
| 59 | + | |
| 60 | + | |
| 61 | + | |
| 62 | + | |
| 63 | + | |
| 64 | + | |
| 65 | + | |
| 66 | + | |
| 67 | + | |
| 68 | + | |
| 69 | + | |
| 70 | + | |
| 71 | + | |
| 72 | + | |
| 73 | + | |
| 74 | + | |
| 75 | + | |
| 76 | + | |
| 77 | + | |
| 78 | + | |
| 79 | + | |
| 80 | + | |
| 81 | + | |
| 82 | + | |
| 83 | + | |
| 84 | + | |
| 85 | + | |
| 86 | + | |
| 87 | + | |
| 88 | + | |
| 89 | + | |
| 90 | + | |
| 91 | + | |
| 92 | + | |
| 93 | + | |
| 94 | + | |
| 95 | + | |
| 96 | + | |
| 97 | + | |
| 98 | + | |
| 99 | + | |
| 100 | + | |
| 101 | + | |
| 102 | + | |
| 103 | + | |
| 104 | + | |
| 105 | + | |
| 106 | + | |
| 107 | + | |
| 108 | + | |
| 109 | + | |
| 110 | + | |
| 111 | + | |
| 112 | + | |
| 113 | + | |
| 114 | + | |
| 115 | + | |
| 116 | + | |
| 117 | + | |
| 118 | + | |
| 119 | + | |
| 120 | + | |
| 121 | + | |
| 122 | + | |
| 123 | + | |
| 124 | + | |
| 125 | + | |
| 126 | + |
+13-14
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
30 | 30 |
| |
31 | 31 |
| |
32 | 32 |
| |
33 |
| - | |
34 |
| - | |
35 |
| - | |
36 |
| - | |
| 33 | + | |
| 34 | + | |
| 35 | + | |
37 | 36 |
| |
38 | 37 |
| |
39 | 38 |
| |
| |||
56 | 55 |
| |
57 | 56 |
| |
58 | 57 |
| |
59 |
| - | |
| 58 | + | |
60 | 59 |
| |
61 | 60 |
| |
62 | 61 |
| |
| |||
129 | 128 |
| |
130 | 129 |
| |
131 | 130 |
| |
132 |
| - | |
133 |
| - | |
134 |
| - | |
| 131 | + | |
| 132 | + | |
| 133 | + | |
135 | 134 |
| |
136 | 135 |
| |
137 | 136 |
| |
| |||
207 | 206 |
| |
208 | 207 |
| |
209 | 208 |
| |
210 |
| - | |
211 |
| - | |
212 |
| - | |
| 209 | + | |
| 210 | + | |
| 211 | + | |
213 | 212 |
| |
214 | 213 |
| |
215 | 214 |
| |
| |||
234 | 233 |
| |
235 | 234 |
| |
236 | 235 |
| |
237 |
| - | |
238 |
| - | |
| 236 | + | |
| 237 | + | |
239 | 238 |
| |
240 | 239 |
| |
241 |
| - | |
| 240 | + | |
242 | 241 |
| |
243 | 242 |
| |
244 | 243 |
| |
|
0 commit comments