Commit 17e8c63
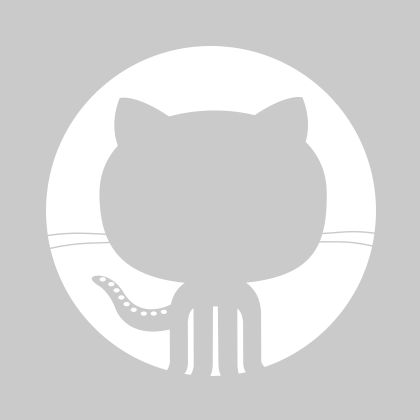
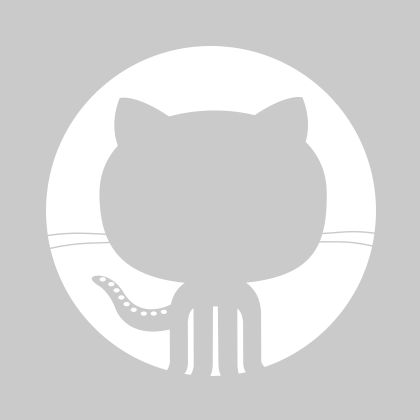
Charlie Frasch
Charlie Frasch
1 parent 69c546a commit 17e8c63
File tree
8 files changed
+181
-15
lines changed8 files changed
+181
-15
lines changedOriginal file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
58 | 58 |
| |
59 | 59 |
| |
60 | 60 |
| |
| 61 | + | |
| 62 | + | |
| 63 | + | |
| 64 | + | |
| 65 | + | |
| 66 | + | |
| 67 | + | |
| 68 | + | |
| 69 | + |
+1-1
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
32 | 32 |
| |
33 | 33 |
| |
34 | 34 |
| |
35 |
| - | |
| 35 | + | |
36 | 36 |
| |
37 | 37 |
| |
38 | 38 |
| |
|
+1-1
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
31 | 31 |
| |
32 | 32 |
| |
33 | 33 |
| |
34 |
| - | |
| 34 | + | |
35 | 35 |
| |
36 | 36 |
| |
37 | 37 |
| |
|
+1-1
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
31 | 31 |
| |
32 | 32 |
| |
33 | 33 |
| |
34 |
| - | |
| 34 | + | |
35 | 35 |
| |
36 | 36 |
| |
37 | 37 |
| |
|
+1-1
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
42 | 42 |
| |
43 | 43 |
| |
44 | 44 |
| |
45 |
| - | |
| 45 | + | |
46 | 46 |
| |
47 | 47 |
| |
48 | 48 |
| |
|
+101
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
| 1 | + | |
| 2 | + | |
| 3 | + | |
| 4 | + | |
| 5 | + | |
| 6 | + | |
| 7 | + | |
| 8 | + | |
| 9 | + | |
| 10 | + | |
| 11 | + | |
| 12 | + | |
| 13 | + | |
| 14 | + | |
| 15 | + | |
| 16 | + | |
| 17 | + | |
| 18 | + | |
| 19 | + | |
| 20 | + | |
| 21 | + | |
| 22 | + | |
| 23 | + | |
| 24 | + | |
| 25 | + | |
| 26 | + | |
| 27 | + | |
| 28 | + | |
| 29 | + | |
| 30 | + | |
| 31 | + | |
| 32 | + | |
| 33 | + | |
| 34 | + | |
| 35 | + | |
| 36 | + | |
| 37 | + | |
| 38 | + | |
| 39 | + | |
| 40 | + | |
| 41 | + | |
| 42 | + | |
| 43 | + | |
| 44 | + | |
| 45 | + | |
| 46 | + | |
| 47 | + | |
| 48 | + | |
| 49 | + | |
| 50 | + | |
| 51 | + | |
| 52 | + | |
| 53 | + | |
| 54 | + | |
| 55 | + | |
| 56 | + | |
| 57 | + | |
| 58 | + | |
| 59 | + | |
| 60 | + | |
| 61 | + | |
| 62 | + | |
| 63 | + | |
| 64 | + | |
| 65 | + | |
| 66 | + | |
| 67 | + | |
| 68 | + | |
| 69 | + | |
| 70 | + | |
| 71 | + | |
| 72 | + | |
| 73 | + | |
| 74 | + | |
| 75 | + | |
| 76 | + | |
| 77 | + | |
| 78 | + | |
| 79 | + | |
| 80 | + | |
| 81 | + | |
| 82 | + | |
| 83 | + | |
| 84 | + | |
| 85 | + | |
| 86 | + | |
| 87 | + | |
| 88 | + | |
| 89 | + | |
| 90 | + | |
| 91 | + | |
| 92 | + | |
| 93 | + | |
| 94 | + | |
| 95 | + | |
| 96 | + | |
| 97 | + | |
| 98 | + | |
| 99 | + | |
| 100 | + | |
| 101 | + |
+17-11
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
30 | 30 |
| |
31 | 31 |
| |
32 | 32 |
| |
33 |
| - | |
| 33 | + | |
| 34 | + | |
34 | 35 |
| |
35 | 36 |
| |
36 | 37 |
| |
37 |
| - | |
38 |
| - | |
39 | 38 |
| |
40 | 39 |
| |
41 | 40 |
| |
| |||
77 | 76 |
| |
78 | 77 |
| |
79 | 78 |
| |
80 |
| - | |
81 |
| - | |
82 |
| - | |
83 |
| - | |
| 79 | + | |
| 80 | + | |
84 | 81 |
| |
85 | 82 |
| |
86 | 83 |
| |
87 | 84 |
| |
88 |
| - | |
89 |
| - | |
| 85 | + | |
| 86 | + | |
90 | 87 |
| |
91 | 88 |
| |
92 | 89 |
| |
93 | 90 |
| |
94 |
| - | |
| 91 | + | |
| 92 | + | |
| 93 | + | |
| 94 | + | |
95 | 95 |
| |
96 |
| - | |
| 96 | + | |
| 97 | + | |
| 98 | + | |
| 99 | + | |
| 100 | + | |
| 101 | + | |
| 102 | + | |
97 | 103 |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
| 1 | + | |
| 2 | + | |
| 3 | + | |
| 4 | + | |
| 5 | + | |
| 6 | + | |
| 7 | + | |
| 8 | + | |
| 9 | + | |
| 10 | + | |
| 11 | + | |
| 12 | + | |
| 13 | + | |
| 14 | + | |
| 15 | + | |
| 16 | + | |
| 17 | + | |
| 18 | + | |
| 19 | + | |
| 20 | + | |
| 21 | + | |
| 22 | + | |
| 23 | + | |
| 24 | + | |
| 25 | + | |
| 26 | + | |
| 27 | + | |
| 28 | + | |
| 29 | + | |
| 30 | + | |
| 31 | + | |
| 32 | + | |
| 33 | + | |
| 34 | + | |
| 35 | + | |
| 36 | + | |
| 37 | + | |
| 38 | + | |
| 39 | + | |
| 40 | + | |
| 41 | + | |
| 42 | + | |
| 43 | + | |
| 44 | + | |
| 45 | + | |
| 46 | + | |
| 47 | + | |
| 48 | + | |
| 49 | + | |
| 50 | + |
0 commit comments