Commit e340ace
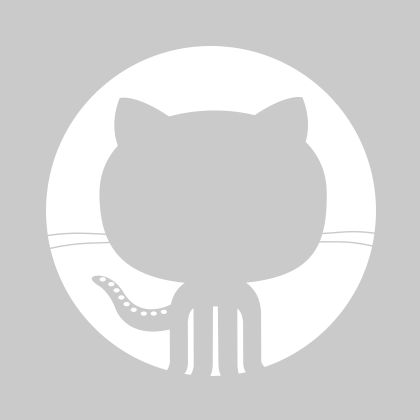
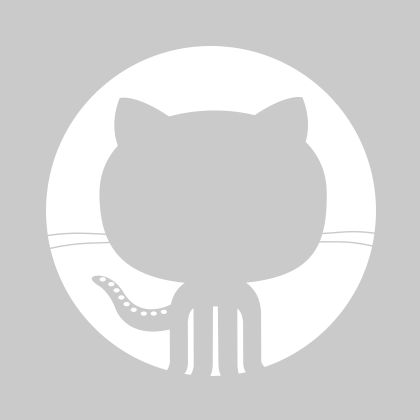
Abraham Rosa Vargas
Abraham Rosa Vargas
1 parent 7d8c77d commit e340ace
File tree
4 files changed
+51
-28
lines changed- src
- __tests__
4 files changed
+51
-28
lines changed+2-2
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
1 | 1 |
| |
2 | 2 |
| |
3 |
| - | |
| 3 | + | |
4 | 4 |
| |
5 | 5 |
| |
6 | 6 |
| |
| |||
177 | 177 |
| |
178 | 178 |
| |
179 | 179 |
| |
180 |
| - | |
| 180 | + |
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
9 | 9 |
| |
10 | 10 |
| |
11 | 11 |
| |
| 12 | + | |
| 13 | + | |
12 | 14 |
| |
13 | 15 |
| |
14 | 16 |
| |
| 17 | + | |
| 18 | + | |
| 19 | + | |
| 20 | + | |
| 21 | + | |
| 22 | + | |
| 23 | + | |
15 | 24 |
| |
16 | 25 |
| |
17 |
| - | |
| 26 | + | |
18 | 27 |
| |
19 | 28 |
| |
20 | 29 |
| |
21 |
| - | |
| 30 | + | |
22 | 31 |
| |
23 | 32 |
| |
24 | 33 |
| |
25 |
| - | |
| 34 | + | |
26 | 35 |
| |
27 | 36 |
| |
28 | 37 |
| |
29 |
| - | |
| 38 | + | |
30 | 39 |
| |
31 | 40 |
| |
32 | 41 |
| |
33 |
| - | |
| 42 | + | |
34 | 43 |
| |
35 | 44 |
| |
36 | 45 |
| |
37 | 46 |
| |
38 | 47 |
| |
39 |
| - | |
| 48 | + | |
40 | 49 |
| |
41 | 50 |
| |
42 | 51 |
| |
43 | 52 |
| |
44 | 53 |
| |
45 |
| - | |
| 54 | + | |
46 | 55 |
| |
47 | 56 |
| |
48 | 57 |
| |
| |||
54 | 63 |
| |
55 | 64 |
| |
56 | 65 |
| |
57 |
| - | |
58 |
| - | |
59 |
| - | |
60 |
| - | |
61 | 66 |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
1 | 1 |
| |
2 | 2 |
| |
3 | 3 |
| |
4 |
| - | |
5 |
| - | |
| 4 | + | |
6 | 5 |
| |
7 | 6 |
| |
8 | 7 |
| |
| |||
33 | 32 |
| |
34 | 33 |
| |
35 | 34 |
| |
36 |
| - | |
37 | 35 |
| |
38 | 36 |
| |
39 | 37 |
| |
| |||
66 | 64 |
| |
67 | 65 |
| |
68 | 66 |
| |
| 67 | + | |
| 68 | + | |
| 69 | + | |
| 70 | + | |
| 71 | + | |
| 72 | + | |
| 73 | + | |
| 74 | + | |
| 75 | + | |
| 76 | + | |
69 | 77 |
| |
70 |
| - | |
71 |
| - | |
| 78 | + | |
| 79 | + | |
| 80 | + | |
| 81 | + | |
| 82 | + | |
| 83 | + | |
72 | 84 |
| |
73 |
| - | |
| 85 | + | |
74 | 86 |
| |
75 | 87 |
| |
76 | 88 |
| |
77 |
| - | |
78 |
| - | |
| 89 | + | |
| 90 | + | |
| 91 | + | |
| 92 | + | |
| 93 | + | |
| 94 | + | |
79 | 95 |
| |
80 | 96 |
| |
81 | 97 |
| |
82 | 98 |
| |
83 |
| - | |
84 |
| - | |
85 |
| - | |
| 99 | + | |
86 | 100 |
| |
87 | 101 |
| |
88 | 102 |
| |
89 |
| - | |
90 |
| - | |
| 103 | + | |
| 104 | + | |
| 105 | + | |
| 106 | + | |
| 107 | + | |
| 108 | + | |
91 | 109 |
| |
92 | 110 |
| |
93 | 111 |
| |
|
+2-2
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
14735 | 14735 |
| |
14736 | 14736 |
| |
14737 | 14737 |
| |
14738 |
| - | |
| 14738 | + | |
14739 | 14739 |
| |
14740 | 14740 |
| |
14741 | 14741 |
| |
| |||
14745 | 14745 |
| |
14746 | 14746 |
| |
14747 | 14747 |
| |
14748 |
| - | |
| 14748 | + | |
14749 | 14749 |
| |
14750 | 14750 |
| |
14751 | 14751 |
| |
|
0 commit comments