|
| 1 | +# Pica OneTool Demo ✨ |
| 2 | + |
| 3 | +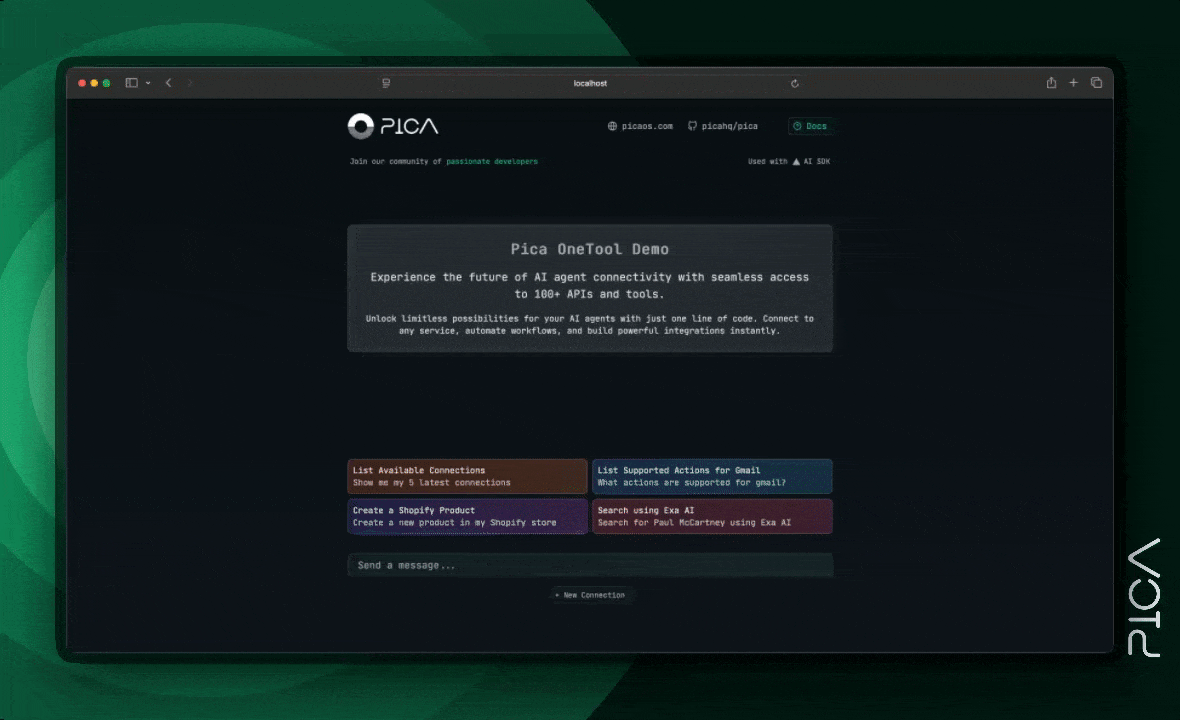 |
| 4 | + |
| 5 | +Experience the future of API integration with [Pica OneTool](https://www.npmjs.com/package/@picahq/ai) - a revolutionary platform that connects you to 100+ APIs and tools with a single line of code. Powered by cutting-edge tech including Next.js 14, TypeScript, Tailwind CSS, Vercel AI SDK, and OpenAI, it's the integration solution you've been dreaming of. |
| 6 | + |
| 7 | +## Overview |
| 8 | + |
| 9 | +Pica OneTool redefines API integration by providing a seamless, AI-powered hub that connects with virtually any service you need. Built on the powerful [@picahq/ai](https://www.npmjs.com/package/@picahq/ai) framework and leveraging the [Vercel AI SDK](https://www.npmjs.com/package/ai) with [OpenAI](https://www.npmjs.com/package/@ai-sdk/openai), it features an intuitive chat interface that lets you execute complex operations across multiple platforms with natural language - no API documentation diving required. Whether you're managing e-commerce platforms, CRM systems, or any other digital service, Pica OneTool handles the complexity so you can focus on what matters. |
| 10 | + |
| 11 | +## Features |
| 12 | + |
| 13 | +- 🔌 **Universal Integration**: Connect to 100+ APIs through a single interface |
| 14 | +- 🤖 **AI-Powered Assistant**: Natural language interface for executing operations |
| 15 | +- 🔄 **CRUD Operations**: Support for all standard operations (Create, Read, Update, Delete) |
| 16 | +- 📊 **Real-time Capabilities**: Dynamic fetching of platform capabilities and requirements |
| 17 | +- 🎨 **Modern UI**: Beautiful, responsive interface with smooth animations and transitions |
| 18 | +- 🔐 **Type-Safe**: Built with TypeScript for robust type checking |
| 19 | +- 📱 **Responsive Design**: Works seamlessly across desktop and mobile devices |
| 20 | +- 🔍 **Tool Observability**: Collapsible sidebar for monitoring tool executions and responses |
| 21 | + |
| 22 | + |
| 23 | +## Core Capabilities |
| 24 | + |
| 25 | +- **Entity Management** |
| 26 | + - List available connections |
| 27 | + - View and manage products |
| 28 | + - Create new items |
| 29 | + - Update existing records |
| 30 | + - Delete records |
| 31 | + - Count entities |
| 32 | + |
| 33 | +- **Platform Integration** |
| 34 | + - Real-time capability checking |
| 35 | + - Required fields validation |
| 36 | + - Platform-specific model mapping |
| 37 | + - Error handling and feedback |
| 38 | + |
| 39 | +## Tech Stack |
| 40 | + |
| 41 | +- **Frontend**: Next.js 14, React 18 |
| 42 | +- **Styling**: Tailwind CSS |
| 43 | +- **Type Safety**: TypeScript |
| 44 | +- **API Integrations**: Pica |
| 45 | +- **State Management**: React Hooks |
| 46 | +- **UI Components**: Custom components with Tailwind |
| 47 | + |
| 48 | +## Getting Started |
| 49 | + |
| 50 | +1. **Clone the repository:** |
| 51 | +```bash |
| 52 | +git clone https://github.com/picahq/onetool-demo.git |
| 53 | +cd onetool-demo |
| 54 | +``` |
| 55 | + |
| 56 | +2. **Install dependencies:** |
| 57 | +```bash |
| 58 | +npm install |
| 59 | +``` |
| 60 | + |
| 61 | +3. **Create a `.env` file in the root directory from the `.env.example` file:** |
| 62 | +```env |
| 63 | +PICA_SECRET_KEY=your_secret_key |
| 64 | +OPENAI_API_KEY=your_openai_api_key |
| 65 | +``` |
| 66 | + |
| 67 | +| Variable | Description | Required | |
| 68 | +|----------|-------------|----------| |
| 69 | +| `PICA_SECRET_KEY` | Your [Pica API secret key](https://app.picaos.com/settings/api-keys) | Yes | |
| 70 | +| `OPENAI_API_KEY` | Your OpenAI API key | Yes | |
| 71 | + |
| 72 | +4. **Run the development server:** |
| 73 | +```bash |
| 74 | +npm run dev |
| 75 | +``` |
| 76 | + |
| 77 | +5. **Open [http://localhost:3000](http://localhost:3000) in your browser 🚀** |
| 78 | + |
| 79 | +## Usage |
| 80 | + |
| 81 | +Here are some example commands you can use to get started: |
| 82 | + |
| 83 | +- What connections are available? |
| 84 | +- What models are supported for Quickbooks? |
| 85 | +- Create a new Shopify product |
| 86 | +- What fields are required for Gmail messages? |
| 87 | +- What caveats are there for Quickbooks Accounts? |
| 88 | +- What actions are supported for Attio contacts? |
| 89 | + |
| 90 | + |
| 91 | +## Support |
| 92 | + |
| 93 | +For support, please visit [picaos.com](https://picaos.com) or contact our support team. |
0 commit comments