Commit fc33980
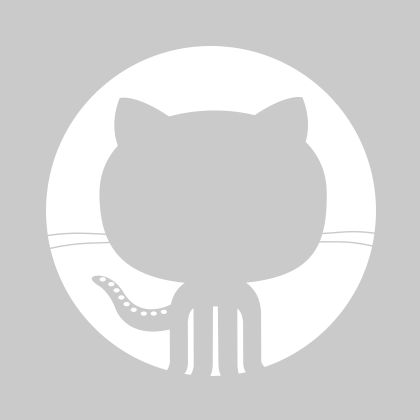
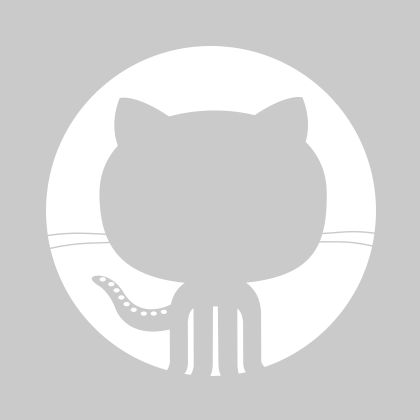
Charlie Frasch
Charlie Frasch
1 parent a395ce8 commit fc33980
File tree
13 files changed
+866
-221
lines changed13 files changed
+866
-221
lines changedLines changed: 21 additions & 39 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
17 | 17 |
| |
18 | 18 |
| |
19 | 19 |
| |
| 20 | + | |
| 21 | + | |
20 | 22 |
| |
21 | 23 |
| |
22 | 24 |
| |
23 | 25 |
| |
24 | 26 |
| |
25 | 27 |
| |
26 | 28 |
| |
27 |
| - | |
28 |
| - | |
29 |
| - | |
30 |
| - | |
31 |
| - | |
32 |
| - | |
33 |
| - | |
34 |
| - | |
35 |
| - | |
36 |
| - | |
37 |
| - | |
38 |
| - | |
39 |
| - | |
40 |
| - | |
41 |
| - | |
42 |
| - | |
43 |
| - | |
44 |
| - | |
45 |
| - | |
46 |
| - | |
47 |
| - | |
48 |
| - | |
49 |
| - | |
50 |
| - | |
51 |
| - | |
52 |
| - | |
53 |
| - | |
54 |
| - | |
55 |
| - | |
56 |
| - | |
57 |
| - | |
58 |
| - | |
59 |
| - | |
60 |
| - | |
61 |
| - | |
62 |
| - | |
63 |
| - | |
64 |
| - | |
65 |
| - | |
| 29 | + | |
| 30 | + | |
| 31 | + | |
| 32 | + | |
| 33 | + | |
| 34 | + | |
| 35 | + | |
| 36 | + | |
| 37 | + | |
| 38 | + | |
| 39 | + | |
| 40 | + | |
| 41 | + | |
| 42 | + | |
| 43 | + | |
| 44 | + | |
| 45 | + | |
| 46 | + | |
| 47 | + | |
66 | 48 |
| |
67 | 49 |
| |
68 | 50 |
| |
|
Lines changed: 29 additions & 20 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
4 | 4 |
| |
5 | 5 |
| |
6 | 6 |
| |
7 |
| - | |
8 |
| - | |
9 |
| - | |
| 7 | + | |
| 8 | + | |
| 9 | + | |
10 | 10 |
| |
11 | 11 |
| |
12 |
| - | |
| 12 | + | |
| 13 | + | |
| 14 | + | |
13 | 15 |
| |
14 |
| - | |
15 |
| - | |
16 |
| - | |
| 16 | + | |
| 17 | + | |
| 18 | + | |
| 19 | + | |
17 | 20 |
| |
18 | 21 |
| |
| 22 | + | |
| 23 | + | |
| 24 | + | |
| 25 | + | |
| 26 | + | |
| 27 | + | |
19 | 28 |
| |
20 | 29 |
| |
21 | 30 |
| |
22 | 31 |
| |
23 | 32 |
| |
| 33 | + | |
24 | 34 |
| |
25 | 35 |
| |
26 |
| - | |
27 |
| - | |
28 |
| - | |
| 36 | + | |
| 37 | + | |
| 38 | + | |
29 | 39 |
| |
30 |
| - | |
| 40 | + | |
| 41 | + | |
| 42 | + | |
31 | 43 |
| |
32 | 44 |
| |
33 | 45 |
| |
| |||
37 | 49 |
| |
38 | 50 |
| |
39 | 51 |
| |
40 |
| - | |
41 |
| - | |
| 52 | + | |
| 53 | + | |
42 | 54 |
| |
43 | 55 |
| |
44 | 56 |
| |
| |||
49 | 61 |
| |
50 | 62 |
| |
51 | 63 |
| |
52 |
| - | |
53 |
| - | |
54 |
| - | |
55 |
| - | |
56 |
| - | |
57 |
| - | |
58 |
| - | |
| 64 | + | |
| 65 | + | |
| 66 | + | |
| 67 | + | |
59 | 68 |
|
Lines changed: 31 additions & 19 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
1 | 1 |
| |
2 | 2 |
| |
3 | 3 |
| |
4 |
| - | |
5 | 4 |
| |
6 | 5 |
| |
7 | 6 |
| |
8 | 7 |
| |
9 |
| - | |
10 |
| - | |
| 8 | + | |
| 9 | + | |
11 | 10 |
| |
12 | 11 |
| |
13 |
| - | |
| 12 | + | |
| 13 | + | |
| 14 | + | |
14 | 15 |
| |
15 |
| - | |
16 |
| - | |
17 |
| - | |
18 |
| - | |
| 16 | + | |
| 17 | + | |
| 18 | + | |
| 19 | + | |
19 | 20 |
| |
20 | 21 |
| |
21 |
| - | |
22 |
| - | |
23 |
| - | |
| 22 | + | |
| 23 | + | |
| 24 | + | |
| 25 | + | |
| 26 | + | |
| 27 | + | |
| 28 | + | |
| 29 | + | |
| 30 | + | |
| 31 | + | |
| 32 | + | |
24 | 33 |
| |
25 |
| - | |
| 34 | + | |
| 35 | + | |
| 36 | + | |
26 | 37 |
| |
27 | 38 |
| |
28 | 39 |
| |
| |||
32 | 43 |
| |
33 | 44 |
| |
34 | 45 |
| |
35 |
| - | |
36 |
| - | |
| 46 | + | |
| 47 | + | |
37 | 48 |
| |
38 | 49 |
| |
39 | 50 |
| |
| |||
44 | 55 |
| |
45 | 56 |
| |
46 | 57 |
| |
47 |
| - | |
| 58 | + | |
| 59 | + | |
48 | 60 |
| |
49 |
| - | |
50 |
| - | |
| 61 | + | |
| 62 | + | |
51 | 63 |
| |
52 | 64 |
| |
53 |
| - | |
| 65 | + | |
54 | 66 |
| |
55 | 67 |
| |
56 |
| - | |
| 68 | + | |
57 | 69 |
|
Lines changed: 40 additions & 22 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
1 | 1 |
| |
2 | 2 |
| |
3 | 3 |
| |
4 |
| - | |
5 | 4 |
| |
6 | 5 |
| |
7 | 6 |
| |
8 | 7 |
| |
9 | 8 |
| |
10 |
| - | |
11 |
| - | |
| 9 | + | |
| 10 | + | |
12 | 11 |
| |
13 | 12 |
| |
14 |
| - | |
| 13 | + | |
| 14 | + | |
| 15 | + | |
15 | 16 |
| |
16 |
| - | |
17 |
| - | |
18 |
| - | |
19 |
| - | |
| 17 | + | |
| 18 | + | |
| 19 | + | |
| 20 | + | |
20 | 21 |
| |
21 | 22 |
| |
22 |
| - | |
23 |
| - | |
24 |
| - | |
| 23 | + | |
| 24 | + | |
| 25 | + | |
| 26 | + | |
| 27 | + | |
| 28 | + | |
| 29 | + | |
| 30 | + | |
| 31 | + | |
| 32 | + | |
| 33 | + | |
25 | 34 |
| |
26 |
| - | |
| 35 | + | |
| 36 | + | |
| 37 | + | |
27 | 38 |
| |
28 | 39 |
| |
29 | 40 |
| |
| |||
35 | 46 |
| |
36 | 47 |
| |
37 | 48 |
| |
38 |
| - | |
39 |
| - | |
| 49 | + | |
| 50 | + | |
40 | 51 |
| |
41 | 52 |
| |
42 | 53 |
| |
| |||
49 | 60 |
| |
50 | 61 |
| |
51 | 62 |
| |
52 |
| - | |
| 63 | + | |
53 | 64 |
| |
54 | 65 |
| |
55 |
| - | |
| 66 | + | |
56 | 67 |
| |
57 | 68 |
| |
58 | 69 |
| |
59 | 70 |
| |
60 |
| - | |
| 71 | + | |
| 72 | + | |
61 | 73 |
| |
62 |
| - | |
63 |
| - | |
| 74 | + | |
| 75 | + | |
64 | 76 |
| |
65 | 77 |
| |
66 |
| - | |
| 78 | + | |
| 79 | + | |
| 80 | + | |
| 81 | + | |
| 82 | + | |
| 83 | + | |
| 84 | + | |
67 | 85 |
| |
68 | 86 |
| |
69 |
| - | |
| 87 | + | |
70 | 88 |
| |
71 | 89 |
| |
72 |
| - | |
| 90 | + | |
73 | 91 |
|
0 commit comments