Commit 4a9c329
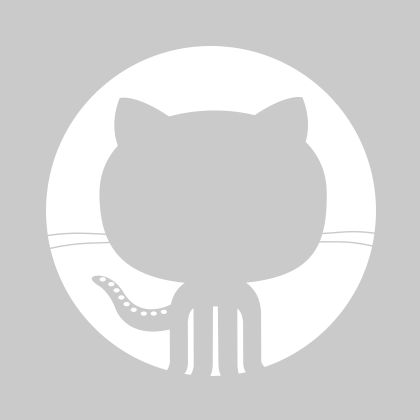
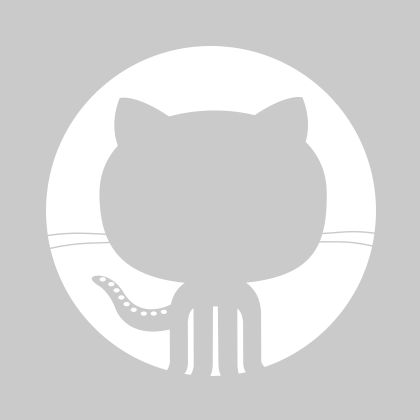
Charlie Frasch
Charlie Frasch
1 parent 8ea1fc3 commit 4a9c329
File tree
8 files changed
+506
-88
lines changed8 files changed
+506
-88
lines changed+26-12
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
1 | 1 |
| |
2 | 2 |
| |
| 3 | + | |
3 | 4 |
| |
4 | 5 |
| |
5 | 6 |
| |
| |||
13 | 14 |
| |
14 | 15 |
| |
15 | 16 |
| |
16 |
| - | |
| 17 | + | |
17 | 18 |
| |
18 |
| - | |
19 |
| - | |
| 19 | + | |
| 20 | + | |
20 | 21 |
| |
21 | 22 |
| |
22 | 23 |
| |
| |||
27 | 28 |
| |
28 | 29 |
| |
29 | 30 |
| |
30 |
| - | |
| 31 | + | |
31 | 32 |
| |
32 | 33 |
| |
33 |
| - | |
| 34 | + | |
34 | 35 |
| |
35 | 36 |
| |
36 |
| - | |
37 |
| - | |
38 |
| - | |
| 37 | + | |
| 38 | + | |
| 39 | + | |
| 40 | + | |
| 41 | + | |
| 42 | + | |
| 43 | + | |
| 44 | + | |
| 45 | + | |
| 46 | + | |
| 47 | + | |
| 48 | + | |
| 49 | + | |
| 50 | + | |
| 51 | + | |
| 52 | + | |
39 | 53 |
| |
40 | 54 |
| |
41 | 55 |
| |
42 | 56 |
| |
43 | 57 |
| |
44 | 58 |
| |
45 | 59 |
| |
46 |
| - | |
| 60 | + | |
47 | 61 |
| |
48 | 62 |
| |
49 | 63 |
| |
| |||
54 | 68 |
| |
55 | 69 |
| |
56 | 70 |
| |
57 |
| - | |
58 |
| - | |
| 71 | + | |
| 72 | + | |
59 | 73 |
| |
60 | 74 |
| |
61 | 75 |
| |
62 | 76 |
| |
63 | 77 |
| |
64 |
| - | |
| 78 | + | |
65 | 79 |
| |
66 | 80 |
| |
67 | 81 |
| |
|
+26-12
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
1 | 1 |
| |
2 | 2 |
| |
3 | 3 |
| |
| 4 | + | |
4 | 5 |
| |
5 | 6 |
| |
6 | 7 |
| |
| |||
13 | 14 |
| |
14 | 15 |
| |
15 | 16 |
| |
16 |
| - | |
| 17 | + | |
17 | 18 |
| |
18 |
| - | |
19 |
| - | |
| 19 | + | |
| 20 | + | |
20 | 21 |
| |
21 | 22 |
| |
22 | 23 |
| |
23 | 24 |
| |
24 |
| - | |
| 25 | + | |
25 | 26 |
| |
26 | 27 |
| |
27 |
| - | |
| 28 | + | |
28 | 29 |
| |
29 | 30 |
| |
30 |
| - | |
31 |
| - | |
32 |
| - | |
| 31 | + | |
| 32 | + | |
| 33 | + | |
| 34 | + | |
| 35 | + | |
| 36 | + | |
| 37 | + | |
| 38 | + | |
| 39 | + | |
| 40 | + | |
| 41 | + | |
| 42 | + | |
| 43 | + | |
| 44 | + | |
| 45 | + | |
| 46 | + | |
33 | 47 |
| |
34 | 48 |
| |
35 | 49 |
| |
36 | 50 |
| |
37 | 51 |
| |
38 | 52 |
| |
39 | 53 |
| |
40 |
| - | |
| 54 | + | |
41 | 55 |
| |
42 | 56 |
| |
43 | 57 |
| |
| |||
48 | 62 |
| |
49 | 63 |
| |
50 | 64 |
| |
51 |
| - | |
52 |
| - | |
| 65 | + | |
| 66 | + | |
53 | 67 |
| |
54 | 68 |
| |
55 | 69 |
| |
56 | 70 |
| |
57 | 71 |
| |
58 |
| - | |
| 72 | + | |
59 | 73 |
| |
60 | 74 |
| |
61 | 75 |
| |
|
+37-18
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
1 | 1 |
| |
2 | 2 |
| |
3 | 3 |
| |
| 4 | + | |
4 | 5 |
| |
5 | 6 |
| |
6 | 7 |
| |
| |||
14 | 15 |
| |
15 | 16 |
| |
16 | 17 |
| |
17 |
| - | |
| 18 | + | |
18 | 19 |
| |
19 |
| - | |
20 |
| - | |
| 20 | + | |
| 21 | + | |
21 | 22 |
| |
22 | 23 |
| |
23 | 24 |
| |
24 | 25 |
| |
25 |
| - | |
| 26 | + | |
26 | 27 |
| |
27 | 28 |
| |
28 |
| - | |
| 29 | + | |
29 | 30 |
| |
30 | 31 |
| |
31 |
| - | |
32 |
| - | |
33 |
| - | |
| 32 | + | |
| 33 | + | |
| 34 | + | |
| 35 | + | |
| 36 | + | |
| 37 | + | |
| 38 | + | |
| 39 | + | |
| 40 | + | |
| 41 | + | |
| 42 | + | |
| 43 | + | |
| 44 | + | |
| 45 | + | |
| 46 | + | |
| 47 | + | |
| 48 | + | |
| 49 | + | |
| 50 | + | |
34 | 51 |
| |
35 | 52 |
| |
36 | 53 |
| |
| |||
40 | 57 |
| |
41 | 58 |
| |
42 | 59 |
| |
43 |
| - | |
| 60 | + | |
44 | 61 |
| |
45 | 62 |
| |
46 | 63 |
| |
| |||
53 | 70 |
| |
54 | 71 |
| |
55 | 72 |
| |
56 |
| - | |
57 |
| - | |
| 73 | + | |
| 74 | + | |
58 | 75 |
| |
59 | 76 |
| |
60 | 77 |
| |
61 | 78 |
| |
62 | 79 |
| |
63 |
| - | |
64 |
| - | |
| 80 | + | |
| 81 | + | |
65 | 82 |
| |
66 |
| - | |
| 83 | + | |
67 | 84 |
| |
68 | 85 |
| |
69 | 86 |
| |
70 | 87 |
| |
71 |
| - | |
| 88 | + | |
72 | 89 |
| |
73 | 90 |
| |
74 | 91 |
| |
75 | 92 |
| |
76 | 93 |
| |
77 |
| - | |
78 |
| - | |
| 94 | + | |
79 | 95 |
| |
80 | 96 |
| |
81 | 97 |
| |
82 | 98 |
| |
83 | 99 |
| |
84 |
| - | |
| 100 | + | |
85 | 101 |
| |
86 | 102 |
| |
87 | 103 |
| |
88 | 104 |
| |
89 | 105 |
| |
90 | 106 |
| |
| 107 | + | |
| 108 | + | |
| 109 | + | |
91 | 110 |
|
+36-17
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
1 | 1 |
| |
2 | 2 |
| |
3 | 3 |
| |
| 4 | + | |
4 | 5 |
| |
5 | 6 |
| |
6 | 7 |
| |
| |||
14 | 15 |
| |
15 | 16 |
| |
16 | 17 |
| |
17 |
| - | |
| 18 | + | |
18 | 19 |
| |
19 |
| - | |
20 |
| - | |
| 20 | + | |
| 21 | + | |
21 | 22 |
| |
22 | 23 |
| |
23 | 24 |
| |
24 | 25 |
| |
25 |
| - | |
| 26 | + | |
26 | 27 |
| |
27 | 28 |
| |
28 |
| - | |
| 29 | + | |
29 | 30 |
| |
30 | 31 |
| |
31 |
| - | |
32 |
| - | |
33 |
| - | |
| 32 | + | |
| 33 | + | |
| 34 | + | |
| 35 | + | |
| 36 | + | |
| 37 | + | |
| 38 | + | |
| 39 | + | |
| 40 | + | |
| 41 | + | |
| 42 | + | |
| 43 | + | |
| 44 | + | |
| 45 | + | |
| 46 | + | |
| 47 | + | |
| 48 | + | |
| 49 | + | |
34 | 50 |
| |
35 | 51 |
| |
36 | 52 |
| |
| |||
43 | 59 |
| |
44 | 60 |
| |
45 | 61 |
| |
46 |
| - | |
| 62 | + | |
47 | 63 |
| |
48 | 64 |
| |
49 | 65 |
| |
| |||
59 | 75 |
| |
60 | 76 |
| |
61 | 77 |
| |
62 |
| - | |
63 |
| - | |
| 78 | + | |
| 79 | + | |
64 | 80 |
| |
65 | 81 |
| |
66 | 82 |
| |
67 | 83 |
| |
68 | 84 |
| |
69 |
| - | |
70 |
| - | |
| 85 | + | |
| 86 | + | |
71 | 87 |
| |
72 |
| - | |
| 88 | + | |
73 | 89 |
| |
74 | 90 |
| |
75 | 91 |
| |
76 | 92 |
| |
77 |
| - | |
| 93 | + | |
78 | 94 |
| |
79 | 95 |
| |
80 | 96 |
| |
81 | 97 |
| |
82 | 98 |
| |
83 |
| - | |
84 |
| - | |
| 99 | + | |
| 100 | + | |
85 | 101 |
| |
86 | 102 |
| |
87 | 103 |
| |
| |||
94 | 110 |
| |
95 | 111 |
| |
96 | 112 |
| |
| 113 | + | |
| 114 | + | |
| 115 | + | |
97 | 116 |
|
0 commit comments