Commit 4cb9f02
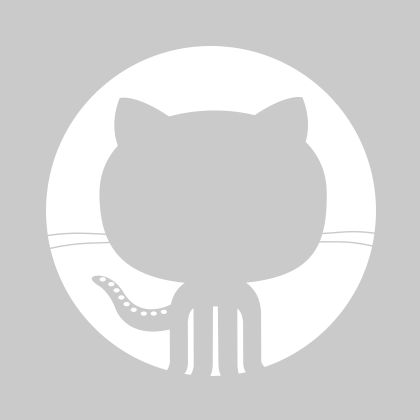
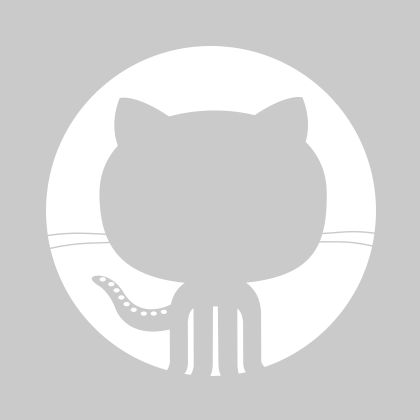
Krishna Kant
Krishna Kant
1 parent c536332 commit 4cb9f02
File tree
8 files changed
+268
-9
lines changed- assets/js
- controllers
- views
8 files changed
+268
-9
lines changedDiff for: assets/js/home_post_comments.js
+100
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
| 1 | + | |
| 2 | + | |
| 3 | + | |
| 4 | + | |
| 5 | + | |
| 6 | + | |
| 7 | + | |
| 8 | + | |
| 9 | + | |
| 10 | + | |
| 11 | + | |
| 12 | + | |
| 13 | + | |
| 14 | + | |
| 15 | + | |
| 16 | + | |
| 17 | + | |
| 18 | + | |
| 19 | + | |
| 20 | + | |
| 21 | + | |
| 22 | + | |
| 23 | + | |
| 24 | + | |
| 25 | + | |
| 26 | + | |
| 27 | + | |
| 28 | + | |
| 29 | + | |
| 30 | + | |
| 31 | + | |
| 32 | + | |
| 33 | + | |
| 34 | + | |
| 35 | + | |
| 36 | + | |
| 37 | + | |
| 38 | + | |
| 39 | + | |
| 40 | + | |
| 41 | + | |
| 42 | + | |
| 43 | + | |
| 44 | + | |
| 45 | + | |
| 46 | + | |
| 47 | + | |
| 48 | + | |
| 49 | + | |
| 50 | + | |
| 51 | + | |
| 52 | + | |
| 53 | + | |
| 54 | + | |
| 55 | + | |
| 56 | + | |
| 57 | + | |
| 58 | + | |
| 59 | + | |
| 60 | + | |
| 61 | + | |
| 62 | + | |
| 63 | + | |
| 64 | + | |
| 65 | + | |
| 66 | + | |
| 67 | + | |
| 68 | + | |
| 69 | + | |
| 70 | + | |
| 71 | + | |
| 72 | + | |
| 73 | + | |
| 74 | + | |
| 75 | + | |
| 76 | + | |
| 77 | + | |
| 78 | + | |
| 79 | + | |
| 80 | + | |
| 81 | + | |
| 82 | + | |
| 83 | + | |
| 84 | + | |
| 85 | + | |
| 86 | + | |
| 87 | + | |
| 88 | + | |
| 89 | + | |
| 90 | + | |
| 91 | + | |
| 92 | + | |
| 93 | + | |
| 94 | + | |
| 95 | + | |
| 96 | + | |
| 97 | + | |
| 98 | + | |
| 99 | + | |
| 100 | + |
Diff for: assets/js/home_posts.js
+117
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
| 1 | + | |
| 2 | + | |
| 3 | + | |
| 4 | + | |
| 5 | + | |
| 6 | + | |
| 7 | + | |
| 8 | + | |
| 9 | + | |
| 10 | + | |
| 11 | + | |
| 12 | + | |
| 13 | + | |
| 14 | + | |
| 15 | + | |
| 16 | + | |
| 17 | + | |
| 18 | + | |
| 19 | + | |
| 20 | + | |
| 21 | + | |
| 22 | + | |
| 23 | + | |
| 24 | + | |
| 25 | + | |
| 26 | + | |
| 27 | + | |
| 28 | + | |
| 29 | + | |
| 30 | + | |
| 31 | + | |
| 32 | + | |
| 33 | + | |
| 34 | + | |
| 35 | + | |
| 36 | + | |
| 37 | + | |
| 38 | + | |
| 39 | + | |
| 40 | + | |
| 41 | + | |
| 42 | + | |
| 43 | + | |
| 44 | + | |
| 45 | + | |
| 46 | + | |
| 47 | + | |
| 48 | + | |
| 49 | + | |
| 50 | + | |
| 51 | + | |
| 52 | + | |
| 53 | + | |
| 54 | + | |
| 55 | + | |
| 56 | + | |
| 57 | + | |
| 58 | + | |
| 59 | + | |
| 60 | + | |
| 61 | + | |
| 62 | + | |
| 63 | + | |
| 64 | + | |
| 65 | + | |
| 66 | + | |
| 67 | + | |
| 68 | + | |
| 69 | + | |
| 70 | + | |
| 71 | + | |
| 72 | + | |
| 73 | + | |
| 74 | + | |
| 75 | + | |
| 76 | + | |
| 77 | + | |
| 78 | + | |
| 79 | + | |
| 80 | + | |
| 81 | + | |
| 82 | + | |
| 83 | + | |
| 84 | + | |
| 85 | + | |
| 86 | + | |
| 87 | + | |
| 88 | + | |
| 89 | + | |
| 90 | + | |
| 91 | + | |
| 92 | + | |
| 93 | + | |
| 94 | + | |
| 95 | + | |
| 96 | + | |
| 97 | + | |
| 98 | + | |
| 99 | + | |
| 100 | + | |
| 101 | + | |
| 102 | + | |
| 103 | + | |
| 104 | + | |
| 105 | + | |
| 106 | + | |
| 107 | + | |
| 108 | + | |
| 109 | + | |
| 110 | + | |
| 111 | + | |
| 112 | + | |
| 113 | + | |
| 114 | + | |
| 115 | + | |
| 116 | + | |
| 117 | + |
Diff for: controllers/commentsController.js
+20-1
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
15 | 15 |
| |
16 | 16 |
| |
17 | 17 |
| |
| 18 | + | |
| 19 | + | |
| 20 | + | |
| 21 | + | |
| 22 | + | |
| 23 | + | |
| 24 | + | |
| 25 | + | |
| 26 | + | |
| 27 | + | |
| 28 | + | |
18 | 29 |
| |
19 | 30 |
| |
20 | 31 |
| |
| |||
36 | 47 |
| |
37 | 48 |
| |
38 | 49 |
| |
39 |
| - | |
| 50 | + | |
| 51 | + | |
| 52 | + | |
| 53 | + | |
| 54 | + | |
| 55 | + | |
| 56 | + | |
| 57 | + | |
| 58 | + | |
40 | 59 |
| |
41 | 60 |
| |
42 | 61 |
| |
|
Diff for: controllers/postsController.js
+20-1
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
4 | 4 |
| |
5 | 5 |
| |
6 | 6 |
| |
7 |
| - | |
| 7 | + | |
8 | 8 |
| |
9 | 9 |
| |
10 | 10 |
| |
11 | 11 |
| |
| 12 | + | |
| 13 | + | |
| 14 | + | |
| 15 | + | |
| 16 | + | |
| 17 | + | |
| 18 | + | |
| 19 | + | |
| 20 | + | |
| 21 | + | |
12 | 22 |
| |
13 | 23 |
| |
14 | 24 |
| |
| |||
28 | 38 |
| |
29 | 39 |
| |
30 | 40 |
| |
| 41 | + | |
| 42 | + | |
| 43 | + | |
| 44 | + | |
| 45 | + | |
| 46 | + | |
| 47 | + | |
| 48 | + | |
| 49 | + | |
31 | 50 |
| |
32 | 51 |
| |
33 | 52 |
| |
|
Diff for: views/_comment.ejs
+1-1
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
1 |
| - | |
| 1 | + | |
2 | 2 |
| |
3 | 3 |
| |
4 | 4 |
| |
|
Diff for: views/_post.ejs
+3-3
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
1 |
| - | |
| 1 | + | |
2 | 2 |
| |
3 | 3 |
| |
4 | 4 |
| |
5 |
| - | |
| 5 | + | |
6 | 6 |
| |
7 | 7 |
| |
8 | 8 |
| |
| |||
14 | 14 |
| |
15 | 15 |
| |
16 | 16 |
| |
17 |
| - | |
| 17 | + | |
18 | 18 |
| |
19 | 19 |
| |
20 | 20 |
| |
|
Diff for: views/home.ejs
+3-3
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
34 | 34 |
| |
35 | 35 |
| |
36 | 36 |
| |
37 |
| - | |
38 |
| - | |
39 |
| - | |
| 37 | + | |
| 38 | + | |
| 39 | + |
Diff for: views/layout.ejs
+4
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
9 | 9 |
| |
10 | 10 |
| |
11 | 11 |
| |
| 12 | + | |
| 13 | + | |
| 14 | + | |
| 15 | + | |
12 | 16 |
| |
13 | 17 |
| |
14 | 18 |
| |
|
0 commit comments