|
| 1 | +# 📙 String Class와 디지털 입력 |
| 2 | + |
| 3 | +## 🌟 String Class |
| 4 | + |
| 5 | +### ✔ String Class |
| 6 | + |
| 7 | +``` |
| 8 | +String string1 = "Hello String"; |
| 9 | +
|
| 10 | +String stringOne = String(13); |
| 11 | +
|
| 12 | +String stringOne = String(45, HEX); //16진법 |
| 13 | +
|
| 14 | +String stringOne = String(5.689, 3); //소숫점 3째자리 |
| 15 | +``` |
| 16 | + |
| 17 | +String("abc") + 123 : (가능) String객체 + 정수 |
| 18 | + |
| 19 | +"abc" + 123 : (불가능) 문자열 + 정수 |
| 20 | + |
| 21 | +<br> |
| 22 | +<br> |
| 23 | + |
| 24 | +### ✔ String Class 함수 |
| 25 | + |
| 26 | +string.compareTo(string2) : 정수로 반환 |
| 27 | + |
| 28 | +string.equals() : 대소문자 구분 -> T/F |
| 29 | + |
| 30 | +string.equlsIgnoreCase : 대소문자 구분X |
| 31 | + |
| 32 | +<br> |
| 33 | +<br> |
| 34 | + |
| 35 | +### ✔ 예시 |
| 36 | + |
| 37 | +- String 객체 생성 및 출력 |
| 38 | + |
| 39 | + ```js |
| 40 | + void setup() { |
| 41 | + Serial.begin(9600); |
| 42 | + } |
| 43 | + |
| 44 | + void loop() { |
| 45 | + String str1 = "One string", str2 = "Another string; |
| 46 | + int n = 1234; |
| 47 | + float f = 3.14; |
| 48 | + char c = 'A'; |
| 49 | +
|
| 50 | + Serial.println() |
| 51 | + Serial.println(str1); // String출력 |
| 52 | + Serial.println(str1 + " " + str2); // 문자열 연결 |
| 53 | + Serial.println(String(n)); // 정수로부 10진 문자열 생성 |
| 54 | + Serial.println(String(n, BIN)); // 정수로부 2진 문자열 생성 |
| 55 | + Serial.println(String(n, HEX)); // 정수로부 16진 문자열 생성 |
| 56 | +
|
| 57 | + Serial.println(String(f)); |
| 58 | + Serial.println(f); |
| 59 | + |
| 60 | + // 다른 데를 연결여 새로운 String 객체를 생성다. |
| 61 | + Serial.println("String + integer : " + String(n)); |
| 62 | + String str3 = "String + character : "; |
| 63 | + str3 += n; |
| 64 | + Serial.println(str3); //Serial.println("String + character : " + 1234) --> 오류!(문자열 + 정수) |
| 65 | + |
| 66 | + while (true); |
| 67 | + } |
| 68 | + ``` |
| 69 | +
|
| 70 | +- Serial로부터 문자열 입력 받기1 |
| 71 | +
|
| 72 | + ```js |
| 73 | + void setup() { |
| 74 | + Serial.begin(9600); // 시리얼 기 |
| 75 | + } |
| 76 | + void loop() { |
| 77 | + int state = 1, len = 0; |
| 78 | + char buffer[128]; |
| 79 | +
|
| 80 | + while (true) { |
| 81 | + if (state == 1) { |
| 82 | + Serial.print("Enter a String --> "); |
| 83 | + state = 2; |
| 84 | + } |
| 85 | + while (Serial.available()) { |
| 86 | + char data = Serial.read(); |
| 87 | + if (data == '\n') { |
| 88 | + // 개 문 ‘\n’ 만날 때까지 읽음 |
| 89 | + buffer[len] = '\0'; |
| 90 | + String in_str = buffer; |
| 91 | + Serial.println(in_str + " [" + in_str.length()+"]"); |
| 92 | + state = 1; |
| 93 | + len = 0; |
| 94 | + break; |
| 95 | + } |
| 96 | + buffer[len++] = data; |
| 97 | + } |
| 98 | + } |
| 99 | + } |
| 100 | + ``` |
| 101 | +
|
| 102 | +- Serial로부터 문자열 입력 받기2 |
| 103 | +
|
| 104 | + ```js |
| 105 | + void setup() { |
| 106 | + Serial.begin(9600); // 시리얼 포트 초기화 |
| 107 | + } |
| 108 | + void loop() { |
| 109 | + int state = 1; |
| 110 | + char buffer[128]; |
| 111 | +
|
| 112 | + while (true) { |
| 113 | + if (state == 1) { |
| 114 | + Serial.print("Enter a String --> "); |
| 115 | + state = 2; |
| 116 | + } |
| 117 | + while (Serial.available()) { |
| 118 | + int len = Serial.readBytesUntil('\n', buffer, 127); |
| 119 | + if (len > 0) { |
| 120 | + buffer[len] = '\0'; |
| 121 | + String in_str = String(buffer); |
| 122 | + Serial.println(in_str + " [" + in_str.length()+"]"); |
| 123 | + state = 1; |
| 124 | + break; |
| 125 | + } |
| 126 | + } |
| 127 | + } |
| 128 | + } |
| 129 | + ``` |
| 130 | +
|
| 131 | +## 🌟 디지털 입력; 버튼 |
| 132 | +
|
| 133 | +Floating Input 문제(1이나 0이 아닌 미결정 상태) 발생 -> 풀다운 저항 사용 |
| 134 | +
|
| 135 | +| <center>풀업/풀다운 저항 사용</center> | <center>버튼off</center> | <center>버튼on</center> | |
| 136 | +|:--------|:--------:|--------:| |
| 137 | +|**사용안함** | <center>플로팅 </center> |*1* | |
| 138 | +|**풀다운** | <center>0 </center> |*1* | |
| 139 | +|**풀업** | <center>1 </center> |*0* | |
| 140 | +
|
| 141 | +
|
| 142 | +### ✔ 풀다운 저항 |
| 143 | +
|
| 144 | +버튼 on : 1 |
| 145 | +버튼 off : 0 |
| 146 | +
|
| 147 | +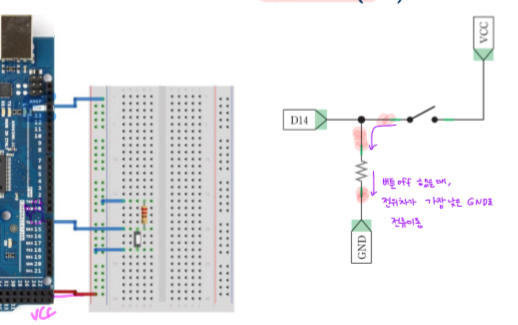 |
| 148 | +
|
| 149 | +<br> |
| 150 | +<br> |
| 151 | +
|
| 152 | +### ✔ 풀업 저항 |
| 153 | +
|
| 154 | +버튼 on : 0 |
| 155 | +버튼 off : 1 |
| 156 | +
|
| 157 | +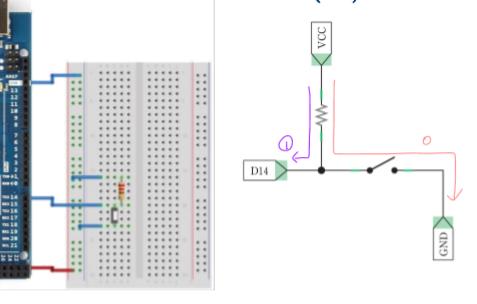 |
| 158 | +
|
| 159 | +```js |
| 160 | +int buttons[] = {14, 15, 16, 17}; // 버튼 연결 핀 |
| 161 | +void setup() { |
| 162 | + Serial.begin(9600); // 시리얼 통신 초기화 |
| 163 | + for (int i = 0; i < 4; i++) { // 버튼 연결 핀을 입력으로 설정 |
| 164 | + pinMode(buttons[i], INPUT); |
| 165 | + } |
| 166 | +} |
| 167 | +void loop() { |
| 168 | + for (int i = 0; i < 4; i++) { |
| 169 | + Serial.print(digitalRead(buttons[i])); // 버튼 상태 출력 |
| 170 | + Serial.print(" "); |
| 171 | + } |
| 172 | + Serial.println(); // 줄바꿈 |
| 173 | + delay(1000); |
| 174 | +} |
| 175 | +
|
| 176 | +``` |
| 177 | +
|
| 178 | +<br> |
| 179 | +<br> |
| 180 | +
|
| 181 | +### ✔ 내장 풀업 저항 사용 |
| 182 | +
|
| 183 | +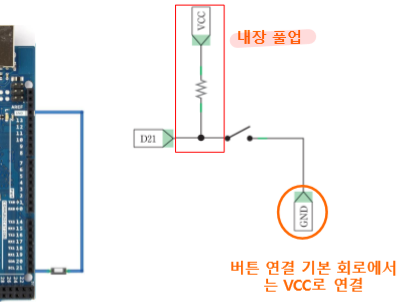 |
| 184 | +
|
| 185 | +```js |
| 186 | +int button = 21; // 버튼 연결 |
| 187 | +void setup() { |
| 188 | + Serial.begin(9600); // 시리얼 통신 초기화 |
| 189 | + pinMode(button, INPUT_PULLUP); // 버튼 연결 핀을 입력으로 설정 |
| 190 | +} |
| 191 | +void loop() { |
| 192 | + Serial.println(digitalRead(button)); // 버튼 상태 출력 |
| 193 | + delay(1000); |
| 194 | +} |
| 195 | +
|
| 196 | +``` |
| 197 | +<br> |
| 198 | +<br> |
| 199 | +
|
| 200 | +### ✔ 버튼 누른 횟수 세기 |
| 201 | +
|
| 202 | +- 버튼을 누른 상태로 있으면 횟수 계속 증가 |
| 203 | +
|
| 204 | +- 이전 상태와 현재 상태 비교 -> 횟수 증가 |
| 205 | +
|
| 206 | +- 바운싱(bouncing) : 기계적 진동에 의해 on/off 반복해서 나타남 |
| 207 | +
|
| 208 | + -> 디바운싱 (delay이용) |
| 209 | +
|
| 210 | + ```js |
| 211 | + int pin_button = 15; // 버튼 연결 핀 |
| 212 | + boolean state_previous = false; // 버튼의 이전 상태 |
| 213 | + boolean state_current; // 버튼의 현재 상태 |
| 214 | + int count = 0; // 버튼 누른 횟수 |
| 215 | + void setup() { |
| 216 | + Serial.begin(9600); // 시리얼 통신 초기화 |
| 217 | + pinMode(pin_button, INPUT); // 버튼 연결 핀을 입력으로 설정 |
| 218 | + } |
| 219 | + void loop() { |
| 220 | + state_current = digitalRead(pin_button); // 버튼 상태 읽기 |
| 221 | + if (state_current) { // 버튼 누른 경우 |
| 222 | + if (state_previous == false) { // 이전 상태와 비교 |
| 223 | + count++; // 상태가 바뀐 경우에만 횟수 증가 |
| 224 | + state_previous = true; |
| 225 | + Serial.println(count); } |
| 226 | +
|
| 227 | + delay(50); // 디바운싱 |
| 228 | + } else { |
| 229 | + state_previous = false; |
| 230 | + } |
| 231 | + } |
| 232 | + } |
| 233 | +
|
| 234 | + ``` |
| 235 | +
|
| 236 | +### 🔎 정리 |
| 237 | +
|
| 238 | +- 데이터 핀을 통해 디지털 데이터 입력 |
| 239 | +
|
| 240 | + pinMode -> digitalRead |
| 241 | +
|
| 242 | +- 플로팅 상태 -> 풀업저항, 풀다운저항, 내장 풀업 저항 |
| 243 | +
|
0 commit comments