Commit a874c0a
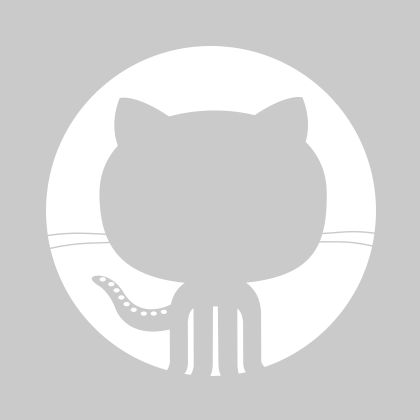
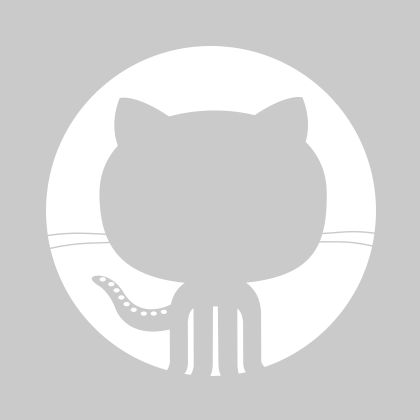
github-actions
github-actions
1 parent b043372 commit a874c0a
File tree
9 files changed
+121
-110
lines changed- src
- main/java/com/examplehub/basics/thread
- test/java/com/examplehub/basics/thread
9 files changed
+121
-110
lines changedLines changed: 28 additions & 28 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
7 | 7 |
| |
8 | 8 |
| |
9 | 9 |
| |
10 |
| - | |
11 |
| - | |
12 |
| - | |
13 |
| - | |
14 |
| - | |
15 |
| - | |
16 |
| - | |
17 |
| - | |
18 |
| - | |
19 |
| - | |
20 |
| - | |
21 |
| - | |
22 |
| - | |
23 |
| - | |
| 10 | + | |
| 11 | + | |
| 12 | + | |
| 13 | + | |
| 14 | + | |
| 15 | + | |
| 16 | + | |
| 17 | + | |
| 18 | + | |
| 19 | + | |
| 20 | + | |
| 21 | + | |
| 22 | + | |
| 23 | + | |
24 | 24 |
| |
25 | 25 |
| |
26 |
| - | |
27 |
| - | |
28 |
| - | |
29 |
| - | |
30 |
| - | |
31 |
| - | |
32 |
| - | |
33 |
| - | |
34 |
| - | |
35 |
| - | |
36 |
| - | |
37 |
| - | |
38 |
| - | |
39 |
| - | |
| 26 | + | |
| 27 | + | |
| 28 | + | |
| 29 | + | |
| 30 | + | |
| 31 | + | |
| 32 | + | |
| 33 | + | |
| 34 | + | |
| 35 | + | |
| 36 | + | |
| 37 | + | |
| 38 | + | |
| 39 | + | |
40 | 40 |
| |
41 | 41 |
| |
42 | 42 |
| |
|
Lines changed: 1 addition & 2 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
1 | 1 |
| |
2 | 2 |
| |
3 |
| - | |
4 |
| - | |
| 3 | + |
Lines changed: 1 addition & 2 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
1 | 1 |
| |
2 | 2 |
| |
3 |
| - | |
4 |
| - | |
| 3 | + |
Lines changed: 1 addition & 2 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
1 | 1 |
| |
2 | 2 |
| |
3 |
| - | |
4 |
| - | |
| 3 | + |
Lines changed: 1 addition & 2 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
1 | 1 |
| |
2 | 2 |
| |
3 |
| - | |
4 |
| - | |
| 3 | + |
Lines changed: 12 additions & 10 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
1 | 1 |
| |
2 | 2 |
| |
3 |
| - | |
| 3 | + | |
4 | 4 |
| |
5 | 5 |
| |
6 | 6 |
| |
7 | 7 |
| |
8 | 8 |
| |
9 |
| - | |
10 |
| - | |
| 9 | + | |
11 | 10 |
| |
12 | 11 |
| |
13 | 12 |
| |
| |||
21 | 20 |
| |
22 | 21 |
| |
23 | 22 |
| |
24 |
| - | |
| 23 | + | |
25 | 24 |
| |
26 | 25 |
| |
27 | 26 |
| |
| |||
30 | 29 |
| |
31 | 30 |
| |
32 | 31 |
| |
33 |
| - | |
| 32 | + | |
34 | 33 |
| |
35 | 34 |
| |
36 | 35 |
| |
37 | 36 |
| |
| 37 | + | |
38 | 38 |
| |
39 | 39 |
| |
40 | 40 |
| |
41 | 41 |
| |
42 | 42 |
| |
43 |
| - | |
44 |
| - | |
45 |
| - | |
46 |
| - | |
| 43 | + | |
| 44 | + | |
| 45 | + | |
| 46 | + | |
| 47 | + | |
| 48 | + | |
47 | 49 |
| |
48 | 50 |
| |
49 | 51 |
| |
50 | 52 |
| |
51 |
| - | |
| 53 | + |
Lines changed: 35 additions & 29 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
1 | 1 |
| |
2 | 2 |
| |
3 |
| - | |
4 |
| - | |
5 | 3 |
| |
6 | 4 |
| |
7 | 5 |
| |
8 | 6 |
| |
9 | 7 |
| |
| 8 | + | |
10 | 9 |
| |
11 | 10 |
| |
12 | 11 |
| |
13 | 12 |
| |
14 | 13 |
| |
15 | 14 |
| |
| 15 | + | |
16 | 16 |
| |
17 | 17 |
| |
18 | 18 |
| |
19 | 19 |
| |
20 | 20 |
| |
21 |
| - | |
| 21 | + | |
22 | 22 |
| |
23 | 23 |
| |
24 | 24 |
| |
| |||
30 | 30 |
| |
31 | 31 |
| |
32 | 32 |
| |
33 |
| - | |
34 |
| - | |
| 33 | + | |
| 34 | + | |
35 | 35 |
| |
36 | 36 |
| |
37 | 37 |
| |
| 38 | + | |
38 | 39 |
| |
39 | 40 |
| |
40 | 41 |
| |
41 | 42 |
| |
42 | 43 |
| |
43 |
| - | |
44 |
| - | |
45 |
| - | |
46 |
| - | |
47 |
| - | |
48 |
| - | |
49 |
| - | |
50 |
| - | |
51 |
| - | |
52 |
| - | |
53 |
| - | |
| 44 | + | |
| 45 | + | |
| 46 | + | |
| 47 | + | |
| 48 | + | |
| 49 | + | |
| 50 | + | |
| 51 | + | |
| 52 | + | |
| 53 | + | |
| 54 | + | |
| 55 | + | |
| 56 | + | |
| 57 | + | |
54 | 58 |
| |
55 | 59 |
| |
56 | 60 |
| |
57 |
| - | |
58 |
| - | |
59 |
| - | |
60 |
| - | |
61 |
| - | |
62 |
| - | |
63 |
| - | |
64 |
| - | |
65 |
| - | |
66 |
| - | |
67 |
| - | |
68 |
| - | |
| 61 | + | |
| 62 | + | |
| 63 | + | |
| 64 | + | |
| 65 | + | |
| 66 | + | |
| 67 | + | |
| 68 | + | |
| 69 | + | |
| 70 | + | |
| 71 | + | |
| 72 | + | |
| 73 | + | |
| 74 | + | |
69 | 75 |
| |
70 | 76 |
| |
71 | 77 |
| |
72 | 78 |
| |
73 | 79 |
| |
74 | 80 |
| |
75 | 81 |
| |
76 |
| - | |
| 82 | + |
Lines changed: 11 additions & 9 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
1 | 1 |
| |
2 | 2 |
| |
3 |
| - | |
| 3 | + | |
4 | 4 |
| |
5 | 5 |
| |
6 | 6 |
| |
7 |
| - | |
8 |
| - | |
| 7 | + | |
9 | 8 |
| |
10 | 9 |
| |
11 | 10 |
| |
12 | 11 |
| |
13 | 12 |
| |
| 13 | + | |
14 | 14 |
| |
15 | 15 |
| |
16 | 16 |
| |
17 | 17 |
| |
18 |
| - | |
| 18 | + | |
19 | 19 |
| |
20 | 20 |
| |
21 | 21 |
| |
| |||
24 | 24 |
| |
25 | 25 |
| |
26 | 26 |
| |
| 27 | + | |
27 | 28 |
| |
28 | 29 |
| |
29 | 30 |
| |
30 | 31 |
| |
31 |
| - | |
32 |
| - | |
33 |
| - | |
34 |
| - | |
| 32 | + | |
| 33 | + | |
| 34 | + | |
| 35 | + | |
| 36 | + | |
35 | 37 |
| |
36 | 38 |
| |
37 | 39 |
| |
38 | 40 |
| |
39 |
| - | |
| 41 | + |
Lines changed: 31 additions & 26 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
1 | 1 |
| |
2 | 2 |
| |
3 |
| - | |
4 |
| - | |
5 | 3 |
| |
6 | 4 |
| |
7 | 5 |
| |
| 6 | + | |
8 | 7 |
| |
9 | 8 |
| |
10 | 9 |
| |
| |||
22 | 21 |
| |
23 | 22 |
| |
24 | 23 |
| |
| 24 | + | |
25 | 25 |
| |
26 | 26 |
| |
27 | 27 |
| |
28 | 28 |
| |
29 | 29 |
| |
30 |
| - | |
31 |
| - | |
32 |
| - | |
33 |
| - | |
34 |
| - | |
35 |
| - | |
36 |
| - | |
37 |
| - | |
38 |
| - | |
39 |
| - | |
40 |
| - | |
| 30 | + | |
| 31 | + | |
| 32 | + | |
| 33 | + | |
| 34 | + | |
| 35 | + | |
| 36 | + | |
| 37 | + | |
| 38 | + | |
| 39 | + | |
| 40 | + | |
| 41 | + | |
| 42 | + | |
| 43 | + | |
41 | 44 |
| |
42 | 45 |
| |
43 | 46 |
| |
44 |
| - | |
45 |
| - | |
46 |
| - | |
47 |
| - | |
48 |
| - | |
49 |
| - | |
50 |
| - | |
51 |
| - | |
52 |
| - | |
53 |
| - | |
54 |
| - | |
55 |
| - | |
| 47 | + | |
| 48 | + | |
| 49 | + | |
| 50 | + | |
| 51 | + | |
| 52 | + | |
| 53 | + | |
| 54 | + | |
| 55 | + | |
| 56 | + | |
| 57 | + | |
| 58 | + | |
| 59 | + | |
| 60 | + | |
56 | 61 |
| |
57 | 62 |
| |
58 | 63 |
| |
59 | 64 |
| |
60 | 65 |
| |
61 | 66 |
| |
62 | 67 |
| |
63 |
| - | |
| 68 | + |
0 commit comments