You signed in with another tab or window. Reload to refresh your session.You signed out in another tab or window. Reload to refresh your session.You switched accounts on another tab or window. Reload to refresh your session.Dismiss alert
CREATE SCHEMA College;
CREATE TABLE students
(
id INT NOT NULL PRIMARY KEY,
Name VARCHAR(20) NOT NULL,
age INT NOT NULL CHECK(age >= 18),
gender VARCHAR(10) NOT NULL ,
phone VARCHAR(10) NOT NULL UNIQUE,
city VARCHAR(20) NOT NULL DEFAULT 'AGRA'
);
INSERT INTO students VALUES
(
(1 , 'Pratap' , 20 , 'male' , '123456789' , 'hazaribagh'),
(2 , 'Ranjeet' , 24 , 'male' , '9876543210' , 'agra'),
(3 , 'Abhishek' , 23 , 'male' , '9087654321', 'ranchi' ),
(4 , 'Deepak' , 24 , 'male' , '897654321' , 'patna'),
(5 , 'Rahul' , 26 , 'male' , '8689754321' , 'hazaribagh')
);# checking for default values
INSERT INTO students(id , name , age , gender , phone) #here we don't add city , however the default value of city can be shown in city
VALUES(6 , 'Vivek' , 24 , 'male', '8976543213');
SELECT * FROM students;
AND , OR , NOT Clause
SELECT*FROM students
WHERE age >=18AND age <=23;
SELECT*FROM students;
SELECT*FROM students
WHERE age >=18AND city ='hazaribagh';
SELECT*FROM students;
SELECT*FROM students
WHERE age >=18OR age <=23;
SELECT*FROM students;
SELECT*FROM students
WHERE NOT city ='hazaribagh';
SELECT*FROM students;
SELECT*FROM students
WHERE NOT city ='hazaribagh'AND age <=23;
SELECT*FROM students;
IN operator
SELECT*FROM students
WHERE age IN (20 , 23); --All students whose age is 20 and 23
SELECT*FROM students
WHERE age NOT IN (20 , 23); --All students whose age is not 20 and 23
SELECT*FROM students
WHERE city IN ('hazaribagh' , 'xyz'); -- All students who are from city hazaribagh and xyz
SELECT*FROM students
WHERE city NOT IN ('hazaribagh' , 'xyz'); --All students who are not from hazaribagh and xyz
Between ans Not Between
SELECT*FROM students
WHERE age BETWEEN 23AND25;
SELECT*FROM students
WHERE age NOT BETWEEN 23AND25;
SELECT*FROM students
WHERE name BETWEEN 'a'AND'r'; -- shows the the record only whose name's first character starts with a and r(including a and excluding r)
SELECT*FROM students
WHERE name NOT BETWEEN 'a'AND'r'; -- shows the the record only whose name's first character does not start with a and r(excluding a and including r)
SELECT*FROM personal INNER JOIN city
ONpersonal.city=city.cid; -- ON means on which column we want to apply inner join### Use aliases to see the records SELECT*FROM personall p INNER JOIN city c -- p and c(alias name) are made short of prersonal and cityONp.city=c.cid;
### Displaying only selected records from tableSELECTp.id,p.name,p.precentage,p.age,p.gender,c.citynameFROM personal p INNER JOIN city c
ONp.city=c.cid;
SELECTp.id,p.name,p.precentage,p.age,p.gender,c.citynameFROM personal p INNER JOIN city c
ONp.city=c.cidWHEREc.cityname='agra';
SELECTp.id,p.name,p.precentage,p.age,p.gender,c.citynameFROM personal p INNER JOIN city c
ONp.city=c.cidWHEREc.cityname='agra'ORDER BY name;
LEFT JOIN Clause
SELECT*FROM personal LEFT JOIN city
ONpersonal.city=city.cid;
RIGHT JOIN Clause
SELECT*FROM personal RIGHT JOIN city
ONpersonal.city=city.cid;
CROSS JOIN Clause
Unlike all the previous three joins cross join does not use the concept of Primary and Foreign Key . CROSS JOINs are used to combine each row of one table with each row of another table, and return the Cartesian product of the # sets of rows from the tables that are joined.
SELECT*FROM personal CROSS JOIN city;
SELECT*FROM presonal , city; -- Prints the same result
MULTIPLE JOINS
CREATETABLEcourses(
course_id INTNOT NULL AUTO INCREMENT
course_name VARCHAR(20)
);
INSERT INTO`college`.`courses` (`course_id`, `course_name`) VALUES ('1', 'B.tech');
INSERT INTO`college`.`courses` (`course_id`, `course_name`) VALUES ('2', 'BCA');
INSERT INTO`college`.`courses` (`course_id`, `course_name`) VALUES ('3', 'MCA');
### NOTE:- ADD a new column in the personal tableALTERTABLE`college`.`personal`
ADD COLUMN `courses`VARCHAR(45) NOT NULL AFTER `city`;
### And Insert values in this columnUPDATE`college`.`personal`SET`courses`='1'WHERE (`id`='1');
UPDATE`college`.`personal`SET`courses`='2'WHERE (`id`='2');
UPDATE`college`.`personal`SET`courses`='1'WHERE (`id`='3');
UPDATE`college`.`personal`SET`courses`='1'WHERE (`id`='4');
UPDATE`college`.`personal`SET`courses`='3'WHERE (`id`='5');
UPDATE`college`.`personal`SET`courses`='2'WHERE (`id`='6');
UPDATE`college`.`personal`SET`courses`='3'WHERE (`id`='7');
### Now apply multiple joinSELECT*FROM personal p
INNER JOIN city c
ONp.city=c.cidINNER JOIN courses cr
ONp.courses=cr.course_id;
GROUP BY & HAVING Clause
GROUP BY
SELECT city , COUNT(city)
FROM personal
GROUP BY city
-----------------------------------------SELECTc.cityname , COUNT(p.city)
FROM personal p INNER JOIN city c
ONp.city=c.cidGROUP BY city;
-----------------------------------------SELECTc.cityname , COUNT(p.city)
FROM personal p INNER JOIN city c
ONp.city=c.cidWHEREp.age>=20--total student whose age is grater equals to 20GROUP BY city;
------------------------------------------SELECTc.cityname , COUNT(p.city)
FROM personal p INNER JOIN city c
ONp.city=c.cidGROUP BY city
ORDER BYCOUNT(p.city) DESC;
-------------------------------------------### HAVING Clause
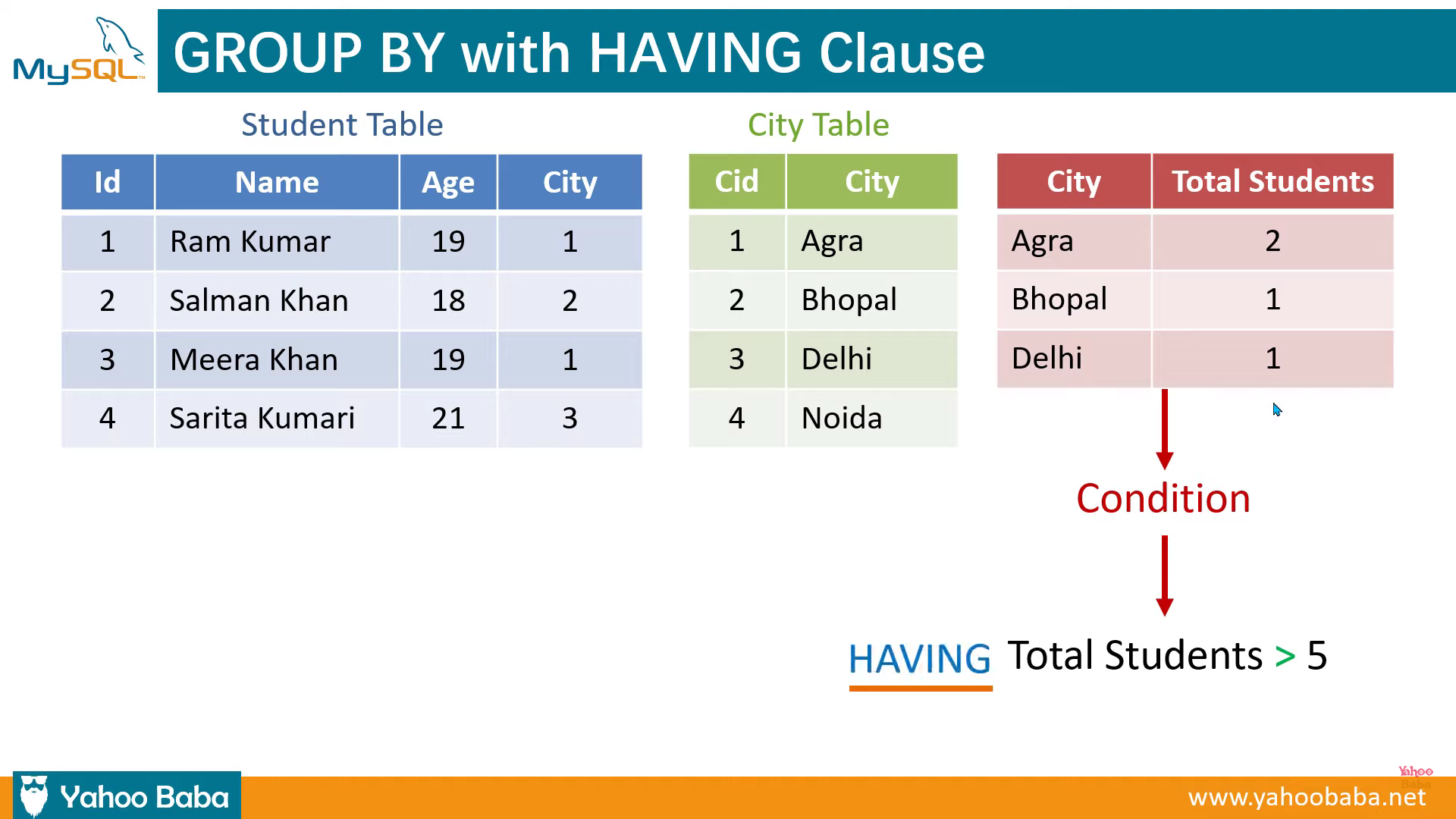
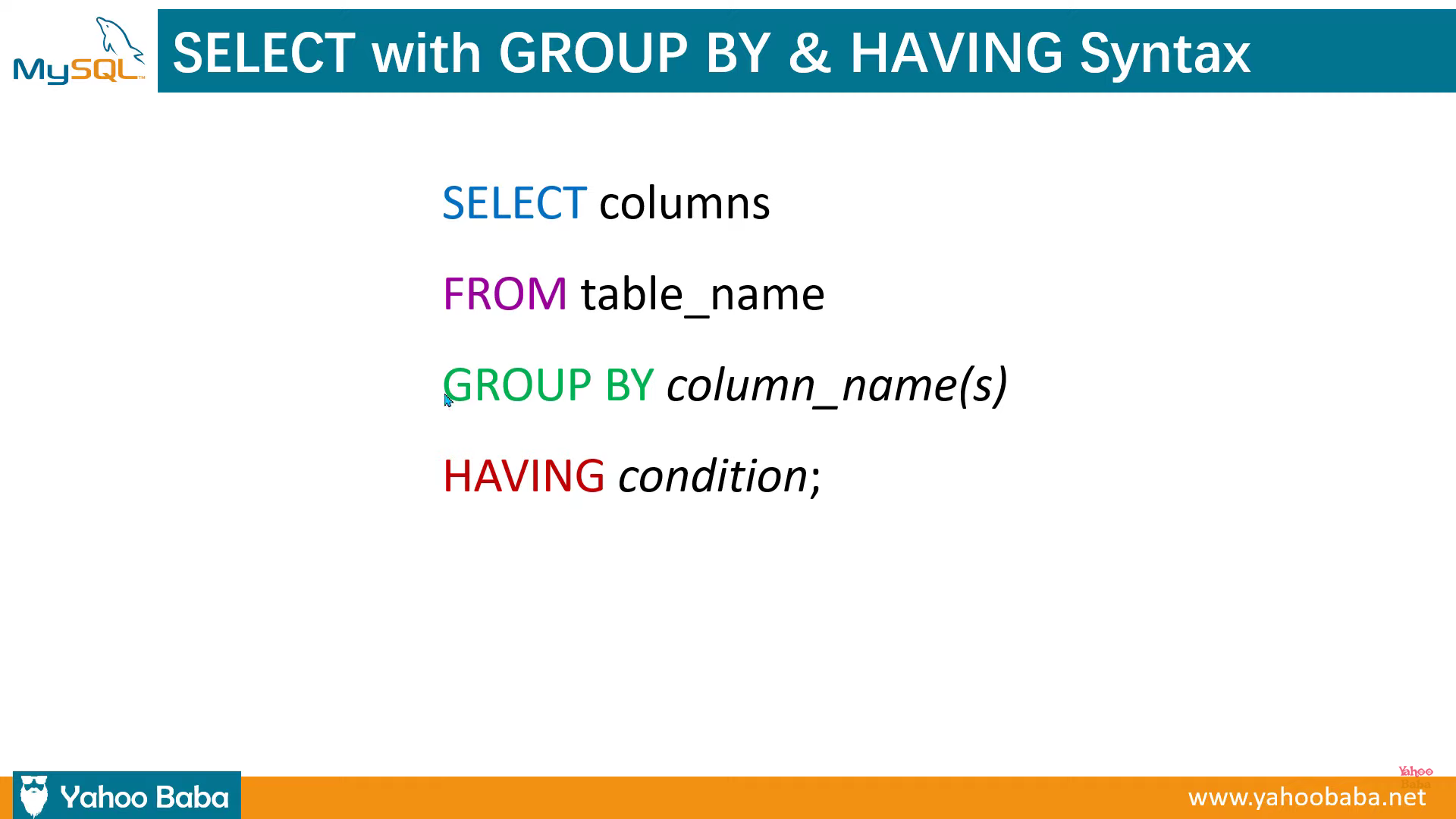
SELECTc.cityname , COUNT(p.city)
FROM personal p INNER JOIN city c
ONp.city=c.cidGROUP BY city -- we apply condition on that result which we obtained from GROUP BYHAVINGCOUNT(p.city) >3 ; -- Name of city where no. of students grater than 3.
SubQuery Or Nested Query (Query inside a query)
SELECT name FROM Personal
WHERE courses = (SELECT course_id FROM courses WHERE course_name="B");
EXISTS And NOT EXISTS
SELECT name FROM Personal
WHERE EXISTS (SELECT course_id FROM courses WHERE course_name="BCA");
-----------------------------------------SELECT name FROM Personal
WHERE NOT EXISTS (SELECT course_id FROM courses WHERE course_name="B.Com");
ALTERTABLE`college`.`students`
ADD COLUMN `percentage`VARCHAR(45) NOT NULL AFTER `gender`;
### Now add value in that columnUPDATE`college`.`students`SET`percentage`='45'WHERE (`id`='1');
UPDATE`college`.`students`SET`percentage`='85'WHERE (`id`='2');
UPDATE`college`.`students`SET`percentage`='29'WHERE (`id`='3');
UPDATE`college`.`students`SET`percentage`='47'WHERE (`id`='4');
UPDATE`college`.`students`SET`percentage`='23'WHERE (`id`='5');
UPDATE`college`.`students`SET`percentage`='45'WHERE (`id`='6');
### The Query Time!!SELECT Id , name , percentage ,
IF(percentage >=33 ,"pass" , "fail") AS Result
FROM students;
### CASE Statment-----------------
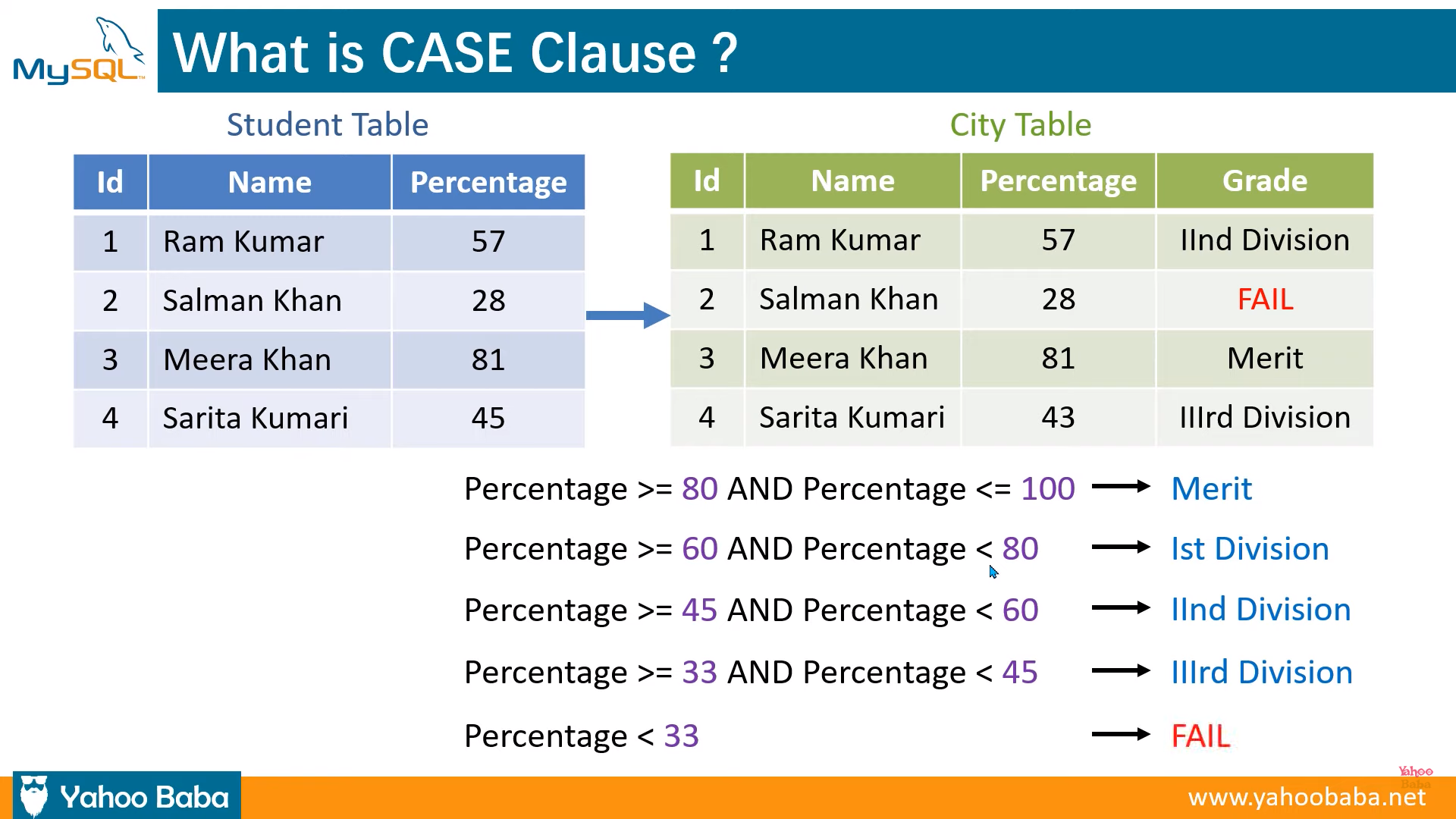
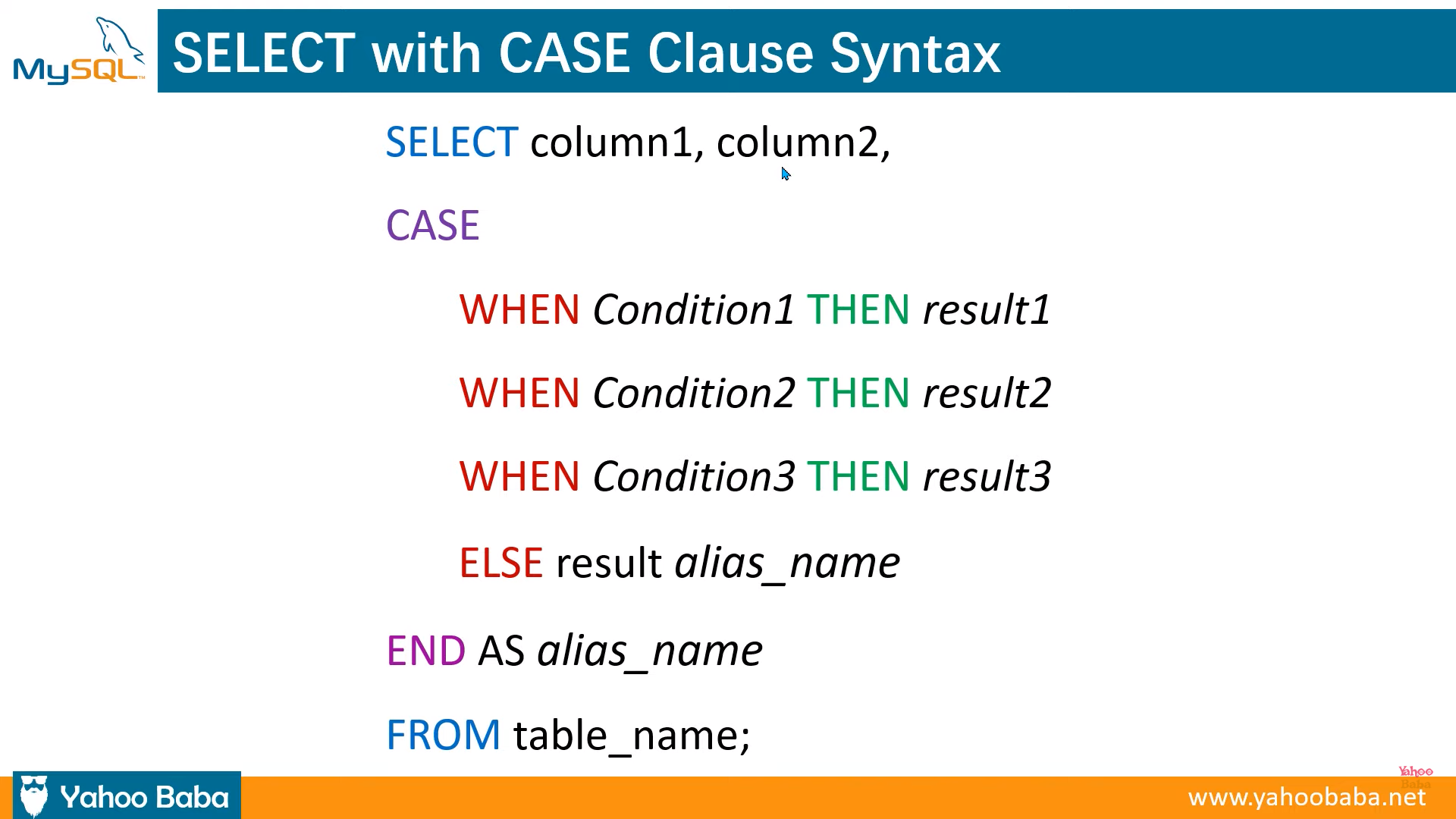
SELECT Id , name , percentage ,
CASE
WHEN percentage >=80AND percentage <=100 THEN "Merit"
WHEN percentage >=60AND percentage <80 THEN "Ist Division"
WHEN percentage >=45AND percentage <60 THEN "IInd Division"
WHEN percentage >=33AND percentage <45 THEN "IIIrd Division"
WHEN percentage <33 THEN "Fail"
ELSE "Invalid %"
END AS Grade
FROM students;
### Updating multiple records at the same timeUPDATE students SET
percentage = (
CASE id
WHEN 3 THEN 64-- when id = 3 set % = 64
WHEN 6 THEN 120-- when id = 7 set % = 120
END
)
WHERE id IN (3 , 6);
MySQL Arithmetic Functions
SELECT id , name , (percentage+5) AS'NEW %'FROM students;
### Some examples SELECT PI() ; -- 3.141593SELECT ROUND(4.51) ; -- 5SELECT ROUND(4.49) ; -- 4SELECT ROUND(-4.49) ; -- -4SELECT ROUND(-4.51) ; -- -5SELECT ROUND(1234.987); -- 1235SELECT ROUND(1234.987,2); -- 1234.99SELECT CEIL(1.36); -- 2SELECT CEIL(1,56); -- 2SELECT FLOOR(4.40) -- 4SELECT FLOOR(4.90) -- 4SELECT POW(2,2) -- 4SELECT POW(2,3) -- 8SELECT SQRT(16) -- 4SELECT SQRT(5) -- 2.23606797749979SELECT ROUND(SQRT(5)) --2SELECT RAND() -- Produces a random no. B/W 0-1 each time we execute the function (with decimal value)SELECT RAND() *100-- Produces a random no. B/W 1-100 each time we execute(with Decimal Value) SELECT ROUND(RAND()*100) -- Produces a random no. B/W 1-100 each time we execute(without Decimal Value) SELECT FLOOR(1+ (RAND()*5)) -- Produces a random no. B/W 1-5 each time we execute(without Decimal Value) SELECT id , name , percentage -- each time we execute the code it the result in different orderFROM students ORDER BY RAND();