diff --git a/CHANGELOG.md b/CHANGELOG.md
index 41cc7d8..a0b6cba 100644
--- a/CHANGELOG.md
+++ b/CHANGELOG.md
@@ -1,3 +1,14 @@
-## 0.0.1
+### 1.1.1
+* [Fix] Better handling of assets
-* TODO: Describe initial release.
+### 1.1.0
+
+* [New] Add custom Giphy selection handler
+
+### 1.0.1
+
+* [Fix] example dependencies
+
+### 1.0.0
+
+* Initial release
diff --git a/README.md b/README.md
index 8415938..7563cb3 100644
--- a/README.md
+++ b/README.md
@@ -1,18 +1,163 @@
-# giphy_selector
+# Giphy Selector
-A new Flutter plugin project.
+[](https://pub.dartlang.org/packages/giphy_selector)
+
+## Overview
+
+Inspired by [giphy_get](https://github.com/bazookon/giphy_get)
+
+This package allow to get gifs, sticker or emojis from [GIPHY](https://www.giphy.com/) in pure dart
+code using [Giphy SDK](https://developers.giphy.com/docs/sdk) design guidelines.
+
+
## Getting Started
-This project is a starting point for a Flutter
-[plug-in package](https://flutter.dev/developing-packages/),
-a specialized package that includes platform-specific implementation code for
-Android and/or iOS.
+Important! you must register your app at [Giphy Develepers](https://developers.giphy.com/dashboard/)
+and get your APIKEY
+
+## Localizations
+
+Currently english, french and spanish is supported.
+
+```dart
+void runApp() {
+ return MaterialApp(
+ title: ' Demo',
+ localizationsDelegates: [
+ // Default Delegates
+ GlobalMaterialLocalizations.delegate,
+ GlobalWidgetsLocalizations.delegate,
+ // Add this line
+ GiphyGetUILocalizations.delegate
+ ],
+ supportedLocales: [
+
+ //Your supported languages
+ Locale('en', ''),
+ Locale('es', ''),
+ Locale('fr', ''),
+ ],
+ home: MyHomePage(title: ' Demo'),
+ );
+}
+```
+
+### Get only Gif
+
+This is for get gif without wrapper and tap to more
+
+```dart
+import 'package:giphy_selector/giphy_selector.dart';
+
+GiphyGif gif = await GiphyGet.getGif(
+ context: context, //Required
+ apiKey: "your api key HERE", //Required.
+ lang: GiphyLanguage.english, //Optional - Language for query.
+ randomID: "abcd", // Optional - An ID/proxy for a specific user.
+ tabColor:Colors.teal, // Optional- default accent color.
+);
+```
+
+### Options
+
+| Value | Type | Description | Default |
+|--------------|--------|------------------------------------------------------------------------------------------------------------------|---------------------------------|
+| `lang` | String | Use [ISO 639-1](https://en.wikipedia.org/wiki/ISO_639-1) language code or use GiphyLanguage constants | `GiphyLanguage.english` |
+| `randomID` | String | An ID/proxy for a specific user. | `null` |
+| `searchText` | String | Input search hint, we recommend use [flutter_18n package](https://pub.dev/packages/flutter_i18n) for translation | `"Search GIPHY"` |
+| `tabColor` | Color | Color for tabs and loading progress, | `Theme.of(context).accentColor` |
+
+### [Get Random ID](https://developers.giphy.com/docs/api/endpoint#random-id)
+
+```dart
+Futurew doSomeThing() async {
+ GiphyClient giphyClient = GiphyClient(apiKey: 'YOUR API KEY');
+ String randomId = await giphyClient.getRandomId();
+}
+```
+
+# Widgets
+
+Optional but this widget is required if you get more gif's of user or view on Giphy following Giphy
+Design guidelines
+
+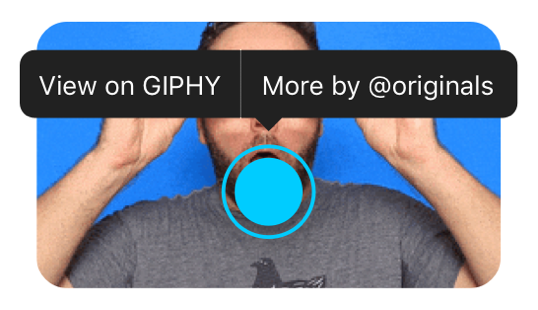
+
+## GiphyGifWidget
+
+Parameters
+
+| Value | Type | Description | Default |
+|----------------------------|-----------------|----------------------------------------------------------------------------------------------------------------------------------------------|------------------|
+| `gif` required | GiphyGif | GiphyGif object from stream or JSON | |
+| `giphyGetWrapper` required | GiphyGetWrapper | selector instance used to find more by author | null |
+| `showGiphyLabel` | bool | show or hide `Powered by GIPHY`label at bottom | true |
+| `borderRadius` | BorderRadius | add border radius to image | null |
+| `imageAlignment` | Alignment | this widget is a [Stack](https://api.flutter.dev/flutter/widgets/Stack-class.html) with Image and tap buttons this property adjust alignment | Alignment.center |
+
+## GiphyGetWrapper
+
+Parameters
+
+| Value | Type | Description | Default |
+|--------------------------|----------|-------------------------------------------------------------|---------|
+| `giphy_api_key` required | String | Your Giphy API KEY | null |
+| `builder` | function | return Stream\ and Instance of GiphyGetWrapper | null |
+
+## Available methods
+
+```dart
+void getGif(String queryText, BuildContext context);
+```
+
+```dart
+void build(BuildContext context) {
+ return GiphyGetWrapper(
+ giphy_api_key: 'REPLACE_WITH YOUR_API_KEY',
+ // Builder with Stream and Instance of GiphyGetWrapper
+ builder: (stream, giphyGetWrapper) =>
+ StreamBuilder(
+ stream: stream,
+ builder: (context, snapshot) {
+ return Scaffold(
+ body: snapshot.hasData
+ ? SizedBox(
+ // GiphyGifWidget with tap to more
+ child: GiphyGifWidget(
+ imageAlignment: Alignment.center,
+ gif: snapshot.data,
+ giphyGetWrapper: giphyGetWrapper,
+ borderRadius: BorderRadius.circular(30),
+ showGiphyLabel: true,
+ ),
+ )
+ : Text("No GIF"),
+ floatingActionButton: FloatingActionButton(
+ onPressed: () async {
+ //Open Giphy Sheet
+ giphyGetWrapper.getGif('', context);
+ },
+ tooltip: 'Open Sticker',
+ child: Icon(Icons.insert_emoticon),
+ ),
+ );
+ }
+ )
+ );
+}
+```
+
+## Using the example
+
+First export your giphy api key
+
+```terminal
+export GIPHY_API_KEY=YOUR_GIPHY_API_KEY
+```
+
+and then run.
-For help getting started with Flutter development, view the
-[online documentation](https://flutter.dev/docs), which offers tutorials,
-samples, guidance on mobile development, and a full API reference.
+## Contrib
-The plugin project was generated without specifying the `--platforms` flag, no platforms are currently supported.
-To add platforms, run `flutter create -t plugin --platforms .` in this directory.
-You can also find a detailed instruction on how to add platforms in the `pubspec.yaml` at https://flutter.dev/docs/development/packages-and-plugins/developing-packages#plugin-platforms.
+Feel free to make any PR's
diff --git a/example/pubspec.yaml b/example/pubspec.yaml
index 6214c2e..5336f5e 100644
--- a/example/pubspec.yaml
+++ b/example/pubspec.yaml
@@ -1,5 +1,5 @@
-name: giphy_get_demo
-description: giphy_get Demo APP.
+name: giphy_selector_demo
+description: giphy_selector Demo APP.
publish_to: "none" # Remove this line if you wish to publish to pub.dev
version: 1.0.1+1
diff --git a/lib/giphy_selector_method_channel.dart b/lib/giphy_selector_method_channel.dart
deleted file mode 100644
index 38ca6d6..0000000
--- a/lib/giphy_selector_method_channel.dart
+++ /dev/null
@@ -1,17 +0,0 @@
-import 'package:flutter/foundation.dart';
-import 'package:flutter/services.dart';
-
-import 'giphy_selector_platform_interface.dart';
-
-/// An implementation of [GiphySelectorPlatform] that uses method channels.
-class MethodChannelGiphySelector extends GiphySelectorPlatform {
- /// The method channel used to interact with the native platform.
- @visibleForTesting
- final methodChannel = const MethodChannel('giphy_selector');
-
- @override
- Future getPlatformVersion() async {
- final version = await methodChannel.invokeMethod('getPlatformVersion');
- return version;
- }
-}
diff --git a/lib/giphy_selector_platform_interface.dart b/lib/giphy_selector_platform_interface.dart
deleted file mode 100644
index 2165ee5..0000000
--- a/lib/giphy_selector_platform_interface.dart
+++ /dev/null
@@ -1,29 +0,0 @@
-import 'package:plugin_platform_interface/plugin_platform_interface.dart';
-
-import 'giphy_selector_method_channel.dart';
-
-abstract class GiphySelectorPlatform extends PlatformInterface {
- /// Constructs a GiphySelectorPlatform.
- GiphySelectorPlatform() : super(token: _token);
-
- static final Object _token = Object();
-
- static GiphySelectorPlatform _instance = MethodChannelGiphySelector();
-
- /// The default instance of [GiphySelectorPlatform] to use.
- ///
- /// Defaults to [MethodChannelGiphySelector].
- static GiphySelectorPlatform get instance => _instance;
-
- /// Platform-specific implementations should set this with their own
- /// platform-specific class that extends [GiphySelectorPlatform] when
- /// they register themselves.
- static set instance(GiphySelectorPlatform instance) {
- PlatformInterface.verifyToken(instance, _token);
- _instance = instance;
- }
-
- Future getPlatformVersion() {
- throw UnimplementedError('platformVersion() has not been implemented.');
- }
-}
diff --git a/pubspec.yaml b/pubspec.yaml
index feaa7d6..5494c83 100644
--- a/pubspec.yaml
+++ b/pubspec.yaml
@@ -1,6 +1,6 @@
name: giphy_selector
description: A Flutter plugin enabling the selection of a GIPHY GIF, Sticker or Emoji
-version: 1.1.0
+version: 1.1.1
homepage: https://github.com/amantoux/giphy_selector
diff --git a/test/giphy_get_test.dart b/test/giphy_get_test.dart
deleted file mode 100644
index 6ba92ba..0000000
--- a/test/giphy_get_test.dart
+++ /dev/null
@@ -1,18 +0,0 @@
-import 'package:flutter/services.dart';
-import 'package:flutter_test/flutter_test.dart';
-
-void main() {
- const MethodChannel channel = MethodChannel('giphy_selector');
-
- TestWidgetsFlutterBinding.ensureInitialized();
-
- setUp(() {
- channel.setMockMethodCallHandler((MethodCall methodCall) async {
- return '42';
- });
- });
-
- tearDown(() {
- channel.setMockMethodCallHandler(null);
- });
-}
diff --git a/test/giphy_selector_method_channel_test.dart b/test/giphy_selector_method_channel_test.dart
deleted file mode 100644
index b5286d9..0000000
--- a/test/giphy_selector_method_channel_test.dart
+++ /dev/null
@@ -1,24 +0,0 @@
-import 'package:flutter/services.dart';
-import 'package:flutter_test/flutter_test.dart';
-import 'package:giphy_selector/giphy_selector_method_channel.dart';
-
-void main() {
- MethodChannelGiphySelector platform = MethodChannelGiphySelector();
- const MethodChannel channel = MethodChannel('giphy_selector');
-
- TestWidgetsFlutterBinding.ensureInitialized();
-
- setUp(() {
- channel.setMockMethodCallHandler((MethodCall methodCall) async {
- return '42';
- });
- });
-
- tearDown(() {
- channel.setMockMethodCallHandler(null);
- });
-
- test('getPlatformVersion', () async {
- expect(await platform.getPlatformVersion(), '42');
- });
-}