Commit 2d8b9d2
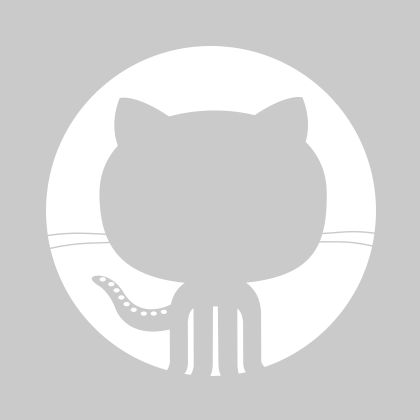
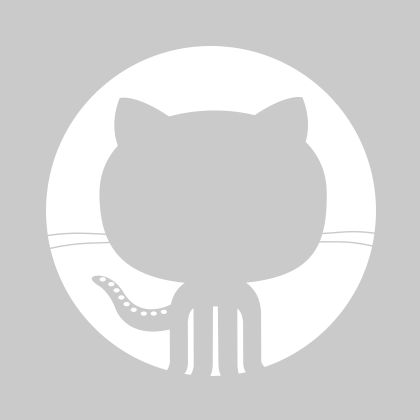
Jaime Salas Zancada
Jaime Salas Zancada
1 parent 0d39ef1 commit 2d8b9d2
File tree
12 files changed
+46
-12
lines changed- 11 Axios
- 03_callbacks
- 05_all
- 06_multiple_params
- 13 Fetch/00_createRequestFactory
- server-mongo
12 files changed
+46
-12
lines changedLines changed: 2 additions & 0 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
60 | 60 |
| |
61 | 61 |
| |
62 | 62 |
| |
| 63 | + | |
| 64 | + |
Lines changed: 1 addition & 1 deletion
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
2 | 2 |
| |
3 | 3 |
| |
4 | 4 |
| |
5 |
| - | |
| 5 | + | |
6 | 6 |
| |
7 | 7 |
| |
8 | 8 |
| |
|
Lines changed: 2 additions & 2 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
5 | 5 |
| |
6 | 6 |
| |
7 | 7 |
| |
8 |
| - | |
| 8 | + | |
9 | 9 |
| |
10 | 10 |
| |
11 |
| - | |
| 11 | + | |
12 | 12 |
| |
13 | 13 |
| |
14 | 14 |
| |
|
Lines changed: 5 additions & 5 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
1 | 1 |
| |
2 | 2 |
| |
3 |
| - | |
| 3 | + | |
4 | 4 |
| |
5 | 5 |
| |
6 | 6 |
| |
| |||
49 | 49 |
| |
50 | 50 |
| |
51 | 51 |
| |
52 |
| - | |
| 52 | + | |
53 | 53 |
| |
54 |
| - | |
| 54 | + | |
55 | 55 |
| |
56 |
| - | |
| 56 | + | |
57 | 57 |
| |
58 |
| - | |
| 58 | + | |
59 | 59 |
| |
60 | 60 |
| |
61 | 61 |
| |
|
Lines changed: 1 addition & 1 deletion
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
141 | 141 |
| |
142 | 142 |
| |
143 | 143 |
| |
144 |
| - | |
| 144 | + | |
145 | 145 |
| |
146 | 146 |
| |
147 | 147 |
| |
|
Lines changed: 9 additions & 0 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
1 | 1 |
| |
2 | 2 |
| |
| 3 | + | |
| 4 | + | |
| 5 | + | |
| 6 | + | |
| 7 | + | |
| 8 | + | |
| 9 | + | |
| 10 | + | |
| 11 | + | |
3 | 12 |
| |
4 | 13 |
| |
5 | 14 |
| |
|
Lines changed: 2 additions & 0 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
| 1 | + | |
| 2 | + |
Lines changed: 9 additions & 0 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
| 1 | + | |
| 2 | + | |
| 3 | + | |
| 4 | + | |
| 5 | + | |
| 6 | + | |
| 7 | + | |
| 8 | + | |
| 9 | + |
Lines changed: 2 additions & 0 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
1 | 1 |
| |
2 | 2 |
| |
3 | 3 |
| |
| 4 | + | |
| 5 | + | |
4 | 6 |
| |
5 | 7 |
| |
6 | 8 |
| |
|
Lines changed: 7 additions & 3 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
3 | 3 |
| |
4 | 4 |
| |
5 | 5 |
| |
6 |
| - | |
| 6 | + | |
| 7 | + | |
| 8 | + | |
7 | 9 |
| |
8 | 10 |
| |
9 | 11 |
| |
| |||
34 | 36 |
| |
35 | 37 |
| |
36 | 38 |
| |
37 |
| - | |
| 39 | + | |
| 40 | + | |
38 | 41 |
| |
39 |
| - | |
| 42 | + | |
| 43 | + | |
40 | 44 |
| |
41 | 45 |
| |
42 | 46 |
| |
|
Lines changed: 5 additions & 0 deletions
Some generated files are not rendered by default. Learn more about customizing how changed files appear on GitHub.
Lines changed: 1 addition & 0 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
12 | 12 |
| |
13 | 13 |
| |
14 | 14 |
| |
| 15 | + | |
15 | 16 |
| |
16 | 17 |
| |
17 | 18 |
| |
|
0 commit comments