Commit 5afd107
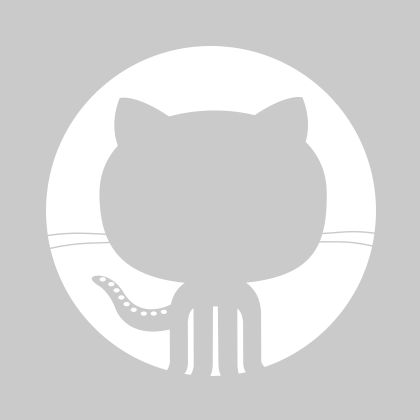
Savina Shen (Manpower Services Taiwan Co Ltd)
1 parent c6e9d9d commit 5afd107
File tree
9 files changed
+94
-47
lines changed- src
- stories
- BasicUsage/MapRef
- DataVisualization
- HeatMapLayer
- ImageLayer
- TileLayer
- DefaultMap
- Events/MapEvents
- MapAnnotations/HtmlMarker
9 files changed
+94
-47
lines changed+8-2
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
4 | 4 |
| |
5 | 5 |
| |
6 | 6 |
| |
7 |
| - | |
8 |
| - | |
| 7 | + | |
| 8 | + | |
| 9 | + | |
| 10 | + | |
| 11 | + | |
| 12 | + | |
| 13 | + | |
| 14 | + | |
9 | 15 |
| |
10 | 16 |
| |
11 | 17 |
| |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
33 | 33 |
| |
34 | 34 |
| |
35 | 35 |
| |
36 |
| - | |
37 |
| - | |
38 |
| - | |
| 36 | + | |
| 37 | + | |
| 38 | + | |
| 39 | + | |
| 40 | + | |
| 41 | + | |
| 42 | + | |
39 | 43 |
| |
40 | 44 |
| |
41 | 45 |
| |
| 46 | + | |
| 47 | + | |
| 48 | + | |
42 | 49 |
| |
43 | 50 |
| |
44 | 51 |
| |
| |||
75 | 82 |
| |
76 | 83 |
| |
77 | 84 |
| |
78 |
| - | |
| 85 | + | |
79 | 86 |
| |
80 | 87 |
| |
81 | 88 |
| |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
7 | 7 |
| |
8 | 8 |
| |
9 | 9 |
| |
10 |
| - | |
11 |
| - | |
| 10 | + | |
| 11 | + | |
12 | 12 |
| |
13 | 13 |
| |
14 | 14 |
| |
| |||
62 | 62 |
| |
63 | 63 |
| |
64 | 64 |
| |
65 |
| - | |
| 65 | + | |
66 | 66 |
| |
67 | 67 |
| |
68 |
| - | |
69 |
| - | |
70 |
| - | |
71 |
| - | |
72 |
| - | |
73 |
| - | |
74 |
| - | |
75 |
| - | |
76 |
| - | |
77 |
| - | |
78 |
| - | |
| 68 | + | |
| 69 | + | |
| 70 | + | |
| 71 | + | |
| 72 | + | |
| 73 | + | |
| 74 | + | |
| 75 | + | |
| 76 | + | |
| 77 | + | |
| 78 | + | |
| 79 | + | |
| 80 | + | |
79 | 81 |
| |
80 | 82 |
| |
81 |
| - | |
82 |
| - | |
83 |
| - | |
84 |
| - | |
85 |
| - | |
86 |
| - | |
87 |
| - | |
88 |
| - | |
89 |
| - | |
90 |
| - | |
91 |
| - | |
92 |
| - | |
93 |
| - | |
94 |
| - | |
95 |
| - | |
| 83 | + | |
| 84 | + | |
| 85 | + | |
| 86 | + | |
| 87 | + | |
| 88 | + | |
| 89 | + | |
| 90 | + | |
| 91 | + | |
| 92 | + | |
| 93 | + | |
| 94 | + | |
96 | 95 |
| |
97 | 96 |
| |
98 | 97 |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
6 | 6 |
| |
7 | 7 |
| |
8 | 8 |
| |
| 9 | + | |
| 10 | + | |
| 11 | + | |
| 12 | + | |
| 13 | + | |
| 14 | + | |
| 15 | + | |
| 16 | + | |
| 17 | + | |
| 18 | + | |
| 19 | + | |
| 20 | + | |
| 21 | + | |
| 22 | + | |
| 23 | + | |
| 24 | + | |
| 25 | + | |
| 26 | + | |
| 27 | + | |
| 28 | + | |
| 29 | + | |
| 30 | + | |
| 31 | + | |
| 32 | + | |
| 33 | + | |
| 34 | + | |
| 35 | + | |
| 36 | + | |
| 37 | + | |
| 38 | + | |
| 39 | + | |
| 40 | + | |
| 41 | + | |
| 42 | + | |
| 43 | + | |
| 44 | + | |
| 45 | + | |
| 46 | + | |
9 | 47 |
| |
10 | 48 |
| |
11 | 49 |
| |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
10 | 10 |
| |
11 | 11 |
| |
12 | 12 |
| |
13 |
| - | |
| 13 | + | |
14 | 14 |
| |
15 | 15 |
| |
16 | 16 |
| |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
29 | 29 |
| |
30 | 30 |
| |
31 | 31 |
| |
32 |
| - | |
33 |
| - | |
34 |
| - | |
35 | 32 |
| |
36 | 33 |
| |
37 | 34 |
| |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
52 | 52 |
| |
53 | 53 |
| |
54 | 54 |
| |
55 |
| - | |
56 |
| - | |
| 55 | + | |
| 56 | + | |
57 | 57 |
| |
58 |
| - | |
59 |
| - | |
| 58 | + | |
| 59 | + | |
60 | 60 |
| |
61 | 61 |
| |
62 | 62 |
| |
| |||
105 | 105 |
| |
106 | 106 |
| |
107 | 107 |
| |
108 |
| - | |
| 108 | + | |
109 | 109 |
| |
110 | 110 |
| |
111 |
| - | |
| 111 | + |
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
20 | 20 |
| |
21 | 21 |
| |
22 | 22 |
| |
| 23 | + | |
23 | 24 |
| |
24 | 25 |
| |
25 | 26 |
| |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
12 | 12 |
| |
13 | 13 |
| |
14 | 14 |
| |
15 |
| - | |
16 | 15 |
| |
17 | 16 |
| |
18 | 17 |
| |
| |||
21 | 20 |
| |
22 | 21 |
| |
23 | 22 |
| |
24 |
| - | |
| 23 | + | |
25 | 24 |
|
0 commit comments